Writing Tests with Spock
Learn about the Spock testing framework by creating a project that uses Spock to unit test Java code.
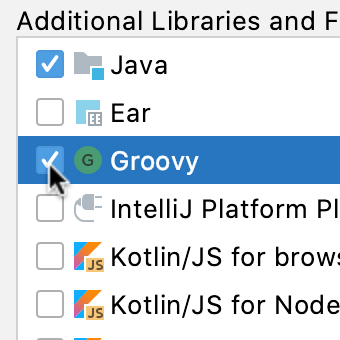
Introduction
Introduction to the tutorial and links to further reading.
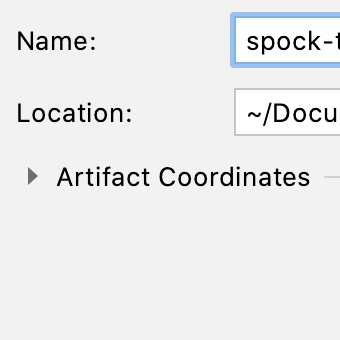
Creating a Project for Spock
Start by creating a new Java project which will be the basis for the rest of this tutorial.
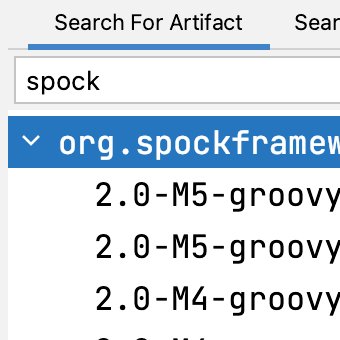
Setting up the Dependencies
Add Spock framework dependencies to the project.
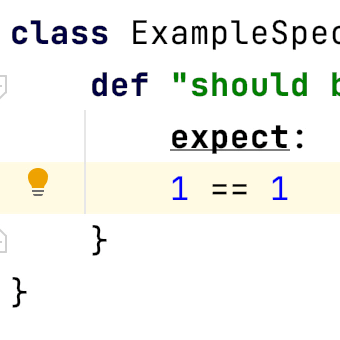
A Simple Assertion
Writing our first Spock test.
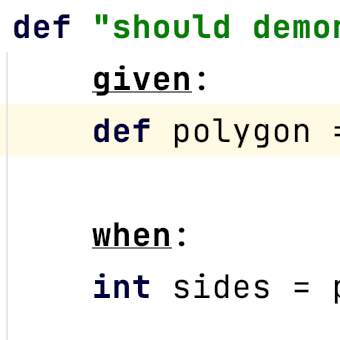
Given, When and Then
See how Spock tests are usually structured.
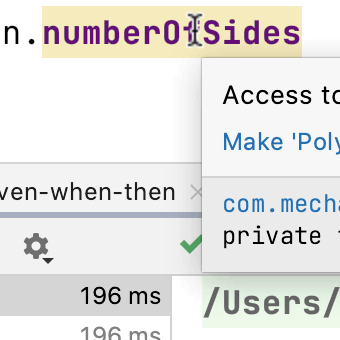
Groovy Tips for Java Developers
Groovy's syntax and functionality might be unusual for Java developers to begin with, but it can be very helpful for testing.
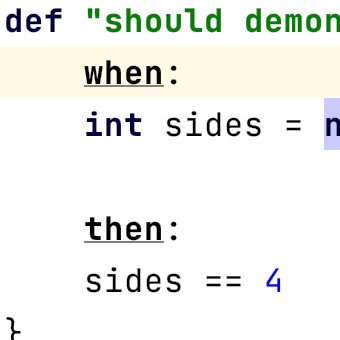
Label Flexibility
Spock supports a range of different test labels to help you write the most readable tests.
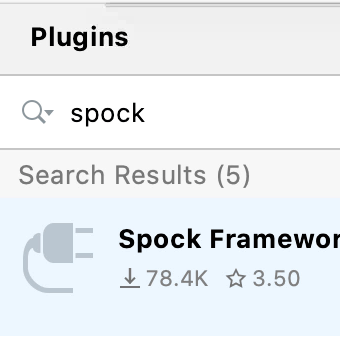
Spock IntelliJ IDEA Plugin
Add the Spock plugin to IntelliJ IDEA to get some extra help in the IDE.
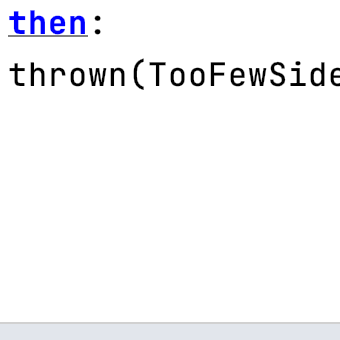
Expecting Exceptions
Tests don't check just the happy paths, sometimes we want to say we're expecting a specific Exception.
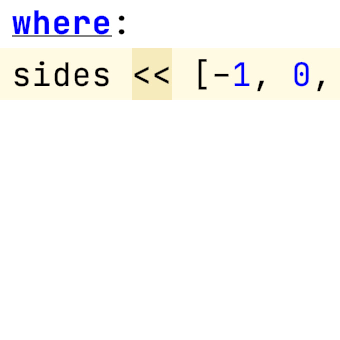
Data Pipes
Often we want to test the same set of criteria with different sets of data. Data pipes is one mechanism to do this.
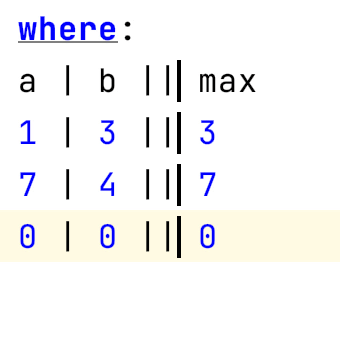
Data Tables
Data tables offer the same functionality as data pipes, but this syntax can sometimes be more readable for more complex sets of data.
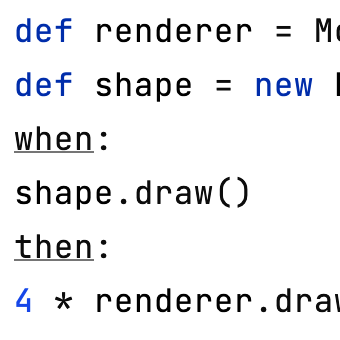
Mocks
We can provide "empty" objects, so we don't have to initialise the whole application to test a section, and we can use mocks to check our code is making the calls we expect.
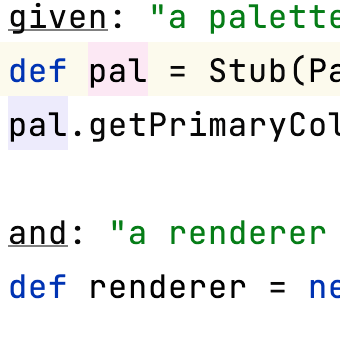
Stubs
Stub can provide simple interfaces, so we don't have to initialise the whole application to test a section.
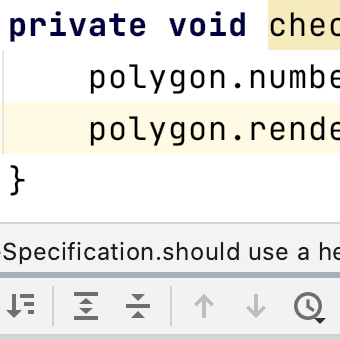
Helper Methods
Sometimes we need to move test code into a separate method. In this section, we look at some tips for this.
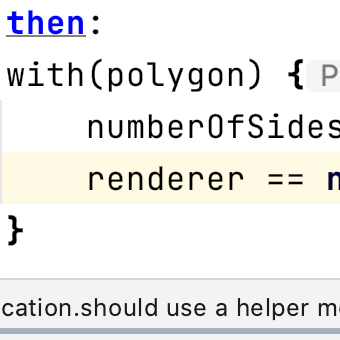
With
Sometimes we want to check more than one value on an object. We can use `with` to do this.
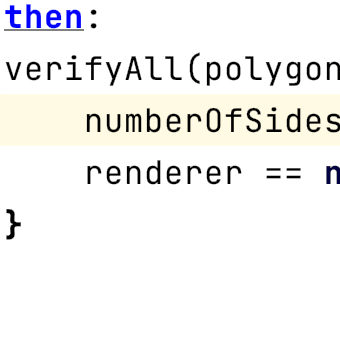
Verify All
Test frameworks often stop the test at the first failure. Find out how to run all conditions to see which pass and which fail.
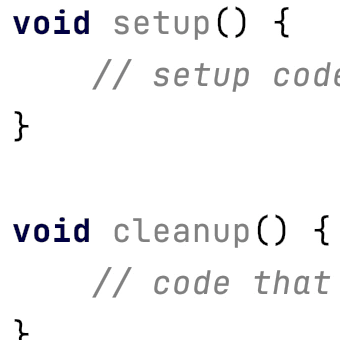
Setup and Teardown
See how to set up, or clean up, test classes and methods in Spock.
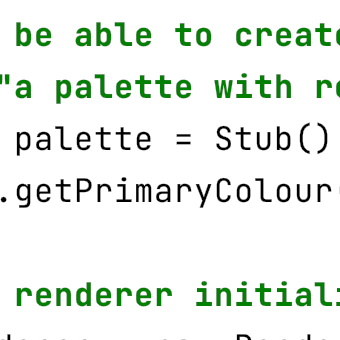
Specifications as Documentation
Spock tests don't just tell the computer how to test the application, they can help developers understand what the application is supposed to do.
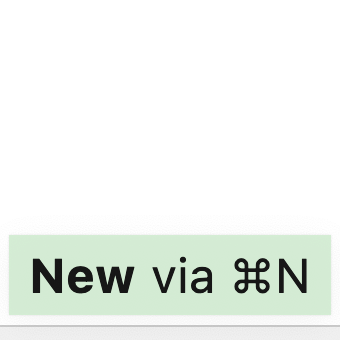
Shortcuts used
Finally, let's summarise the shortcuts we've learnt and see links to further information.