Developing FastAPI Application using K8s & AWS
Build seamless FastAPI applications in PyCharm through Kubernetes & AWS.
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints.
This tutorial will be entirely focused on FastAPI along-with playing with titans like Kubernetes & Amazon Web Services.
There are a lot of features we are going to cover:
- Working with SQLAlchemy & Alembic
- Implementing APIs along-with securing with JSON Web Tokens (JWT)
- Integrating with Celery & Redis
- Async Tests
- Helm Charts
- Deploying Apps using Kubernetes & AWS EKS (Elastic Kubernetes Service)
Note: We'll be using PyCharm Professional Edition for all the steps in this tutorial.
Introduction
Introducing FastAPI, a modern, fast (high-performance), web framework.
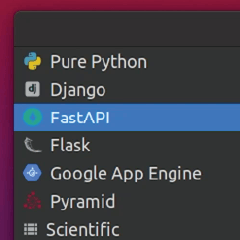
Project Setup
Setting up FastAPI project type in PyCharm Professional.
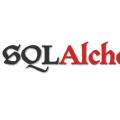
Database Setup - Part I
Configuring database connection with SQLAlchemy and FastAPI.
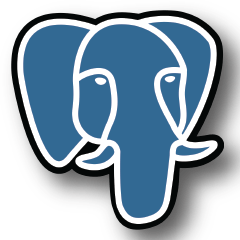
Database Setup - Part II
Setting up database migrations with Alembic.
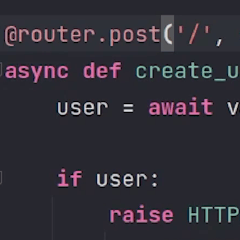
REST API - Part I
Implementing a REST API for User modules using Pydantic & API Router.
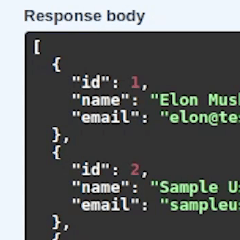
REST API - Part II
Performing CRUD operations in our User module.
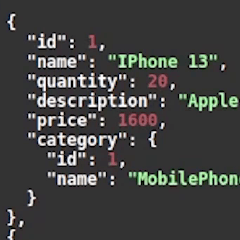
REST API - Part III
Performing CRUD operations in Products & Category Module.
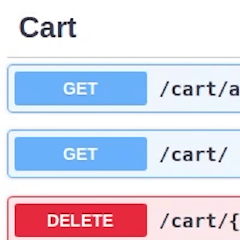
REST API - Part IV
Performing CRUD operations in Orders & Cart Module.
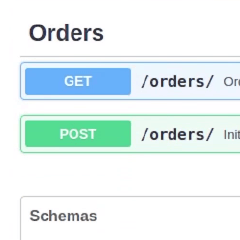
REST API - Part V
Performing CRUD operations in Orders along with placing a new order.
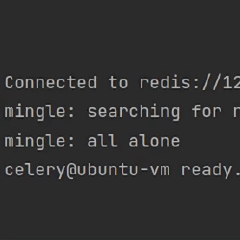
Celery & Redis
Configuring Celery & Redis with FastAPI.
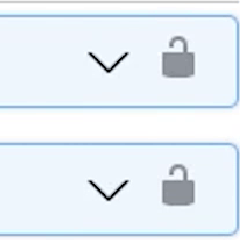
Authentication
Integrating FastAPI with JWT Tokens.
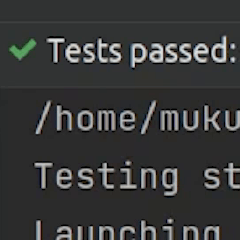
Testing
Writing Unit Tests using Pytest & Asyncio.
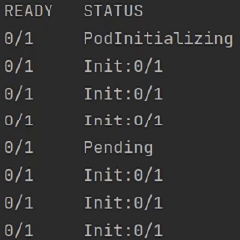
Kubernetes Deployment
Writing K8s manifests & deploying in minikube.
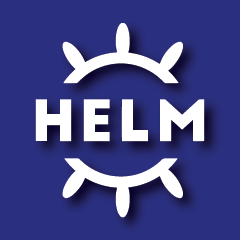
Helm Charts
Combine your K8s manifests into a single package using Helm Charts.
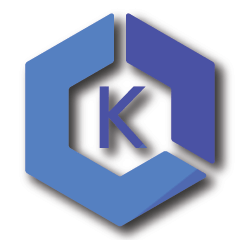
Elastic Kubernetes Service (EKS)
Deploying application using EKS, RDS, ElastiCache, Route53 & AWS Certificate Manager.
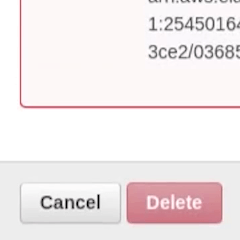
Cleanup
Cleaning up stack (removing RDS, EKS, ElastiCache, LoadBalancer, Route53).
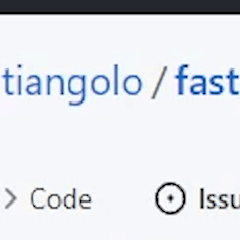
References
Reference materials which helped to prepare this tutorial.