Modify the project
Let's modify the code generated by the Kotlin Multiplatform wizard and display the current date within the App
composable. To do this, you'll add a new dependency to the project, enhance the UI, and rerun the application on each platform.
Add a new dependency
You could retrieve the date using platform-specific libraries and expected and actual declarations. But we recommend that you use this approach only when there's no Kotlin Multiplatform library available. In this case, you can rely on the kotlinx-datetime library.
To use this library:
Open the
composeApp/build.gradle.kts
file and add it as a dependency to the project.commonMain.dependencies { // ... implementation("org.jetbrains.kotlinx:kotlinx-datetime:0.6.0") }The dependency is added to the section that configures the common code source set. With a multiplatform library, you don't need to modify platform-specific source sets.
For simplicity, the version number is included directly instead of being added to the version catalog.
Once the dependency is added, you're prompted to resync the project. Click Sync Now to synchronize Gradle files:
Enhance the user interface
Open the
App.kt
file and add the following function:fun todaysDate(): String { fun LocalDateTime.format() = toString().substringBefore('T') val now = Clock.System.now() val zone = TimeZone.currentSystemDefault() return now.toLocalDateTime(zone).format() }It builds a string containing the current date.
Modify the
App
composable to include theText
composable that invokes this function and displays the result:@Composable @Preview fun App() { MaterialTheme { var showContent by remember { mutableStateOf(false) } val greeting = remember { Greeting().greet() } Column(Modifier.fillMaxWidth(), horizontalAlignment = Alignment.CenterHorizontally) { Text( text = "Today's date is ${todaysDate()}", modifier = Modifier.padding(20.dp), fontSize = 24.sp, textAlign = TextAlign.Center ) Button(onClick = { showContent = !showContent }) { Text("Click me!") } AnimatedVisibility(showContent) { Column(Modifier.fillMaxWidth(), horizontalAlignment = Alignment.CenterHorizontally) { Image(painterResource(Res.drawable.compose_multiplatform), null) Text("Compose: $greeting") } } } } }Follow the IDE's suggestions to import the missing dependencies.
Rerun the application
You can now rerun the application using the same run configurations for Android, iOS, and desktop:
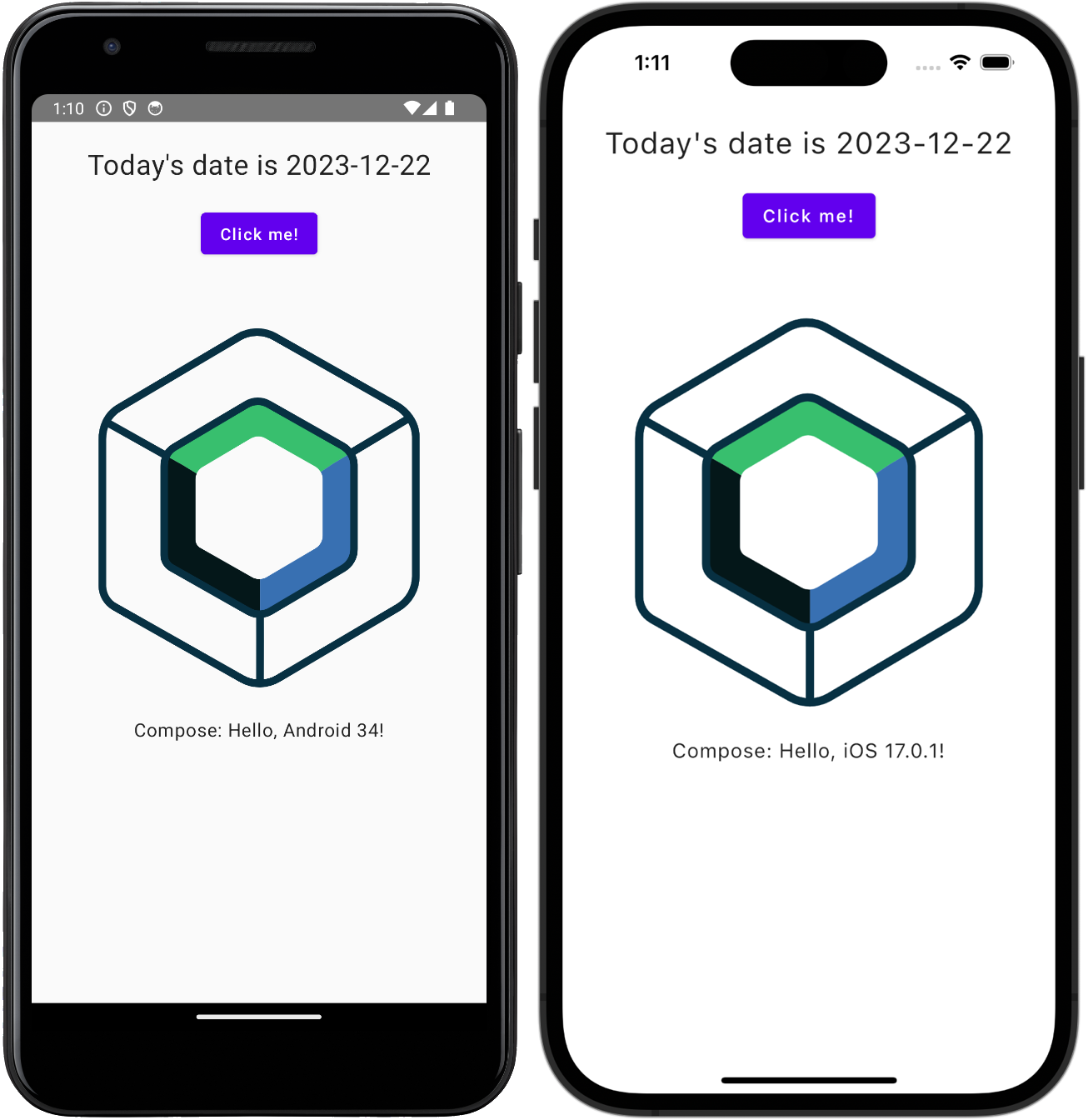
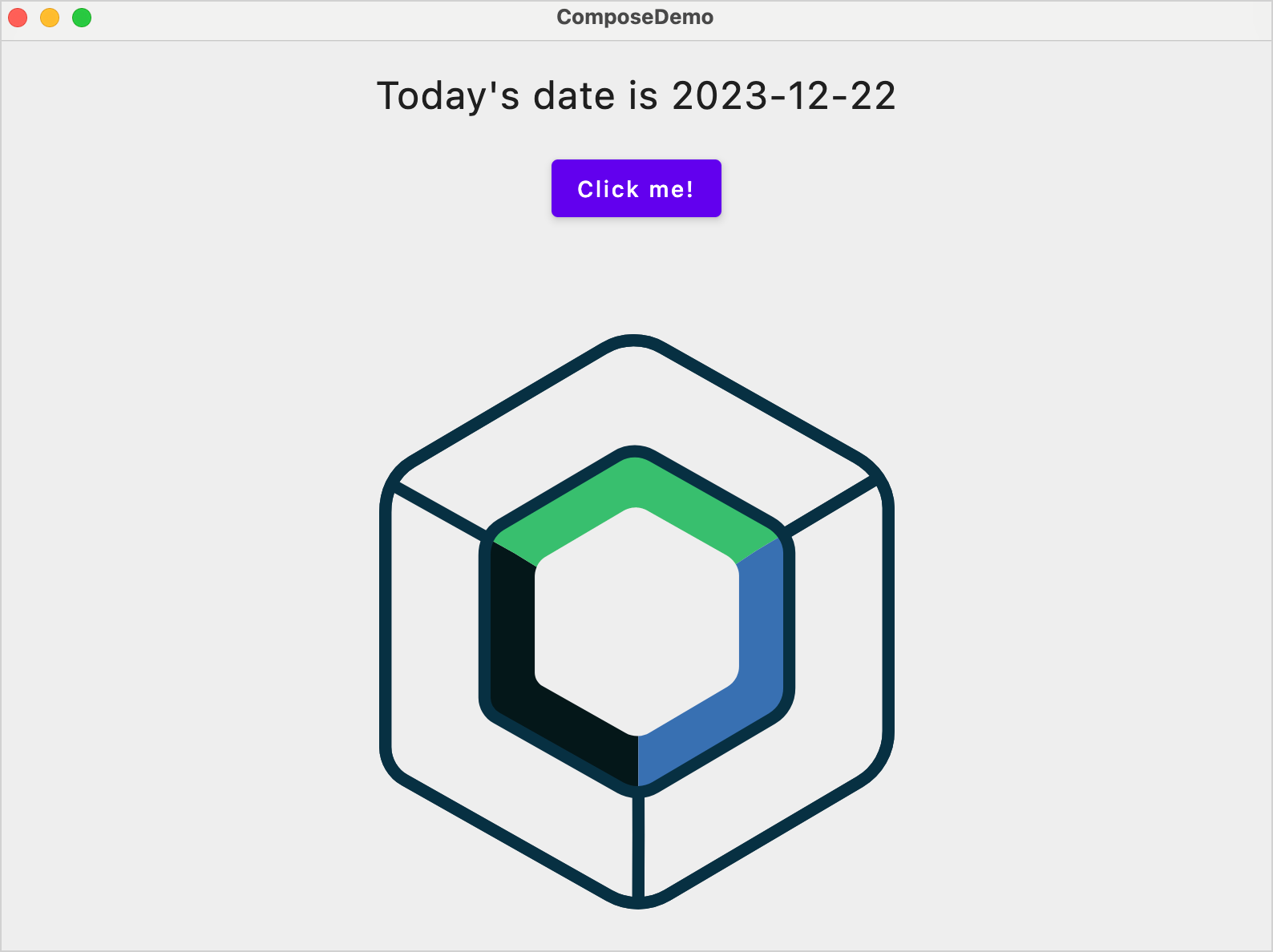
Next step
In the next part of the tutorial, you'll learn new Compose Multiplatform concepts and create your own application from scratch.
Get help
Kotlin Slack. Get an invite and join the #multiplatform channel.
Kotlin issue tracker. Report a new issue.