Create a decorator
The Decorator design pattern is used to dynamically add additional behavior to an object. In addition, by using interfaces, a decorator can be used to unify types in a manner similar to multiple inheritance.
Let’s take an example – suppose you have two objects, called Bird
and Lizard
, that you want to put into a Decorator:
1. Get object interfaces with the extract interface refactoring
Since a decorator cannot be inheriting from both of these classes, we can invoke the Extract Interface refactoring from the Refactor this menu Control+Shift+R for both of these objects to get their interfaces:
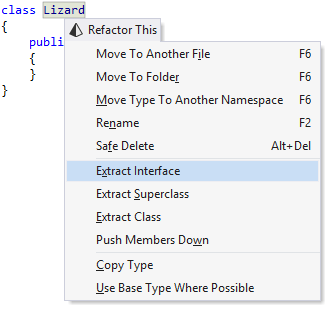
Calling this refactoring pops up a window asking us which members should appear in the interface:
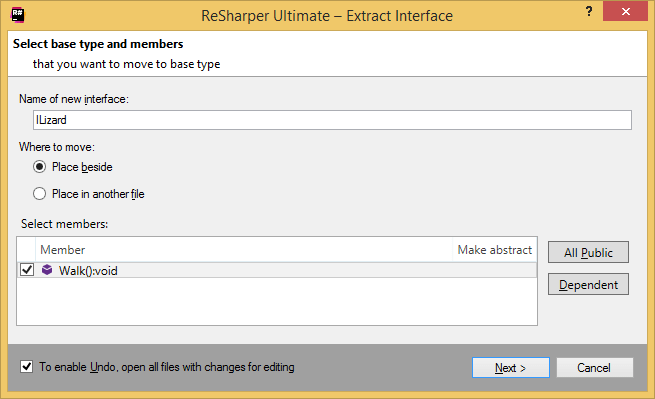
After we do this for both our classes, we end up with the following code:
2. Declare a decorator class
With these interfaces, we can make a decorator. First, we declare a class called Dragon
, indicating that it implements both IBird
and ILizard
:
Now for the aggregated members. The easiest thing to do is to declare both of these as fields first, that is:
3. Use quick-fix to initialize unused fields
Now, we can use apply a quick-fix to unused fields and initialize both of them from constructor parameters:
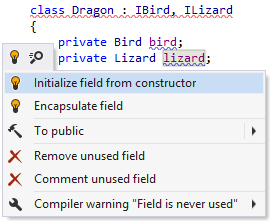
After this, our class will look as follows:
4. Generate delegating members for source objects.
And now for the finishing touch – we want to generate delegating members for both bird and lizard. This is easy — we open the Generate menu Alt+Insert and choose Delegating Members:
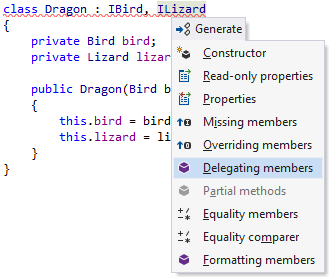
ReSharper then asks us which members we need to delegate:
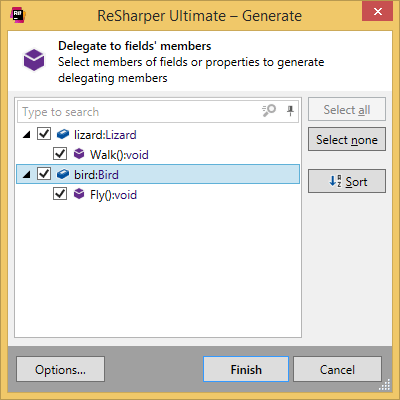
And here is the end result:
That’s it! Our decorator is ready.