Code Templates in C++
All JetBrains Rider's code template features are also supported in C++. You can find the detailed information on these features in the corresponding topics of the .NET-specific templates section.
Live Templates
Live/Surround templates can be used for generating code in existing files and (if there is the $SELECTION$
parameter in the template body) for surrounding code fragments with template code, such as try...catch
statement.
Each live template is identified by a Shortcut — a short string of characters, for example foreach
— that you can type right in the editor to invoke the template.
JetBrains Rider provides plenty of predefined live templates. You can also create custom live templates on the page of JetBrains Rider settings Control+Alt+S.
File Templates
File templates are used to create one or more new files with predefined code, such as a type declaration, unit test, and so on. File templates have no shortcuts, they are identified by their descriptions. For more information, refer to Create files from templates .
There are predefined templates for class, struct, source file and header file. You can also create new file.
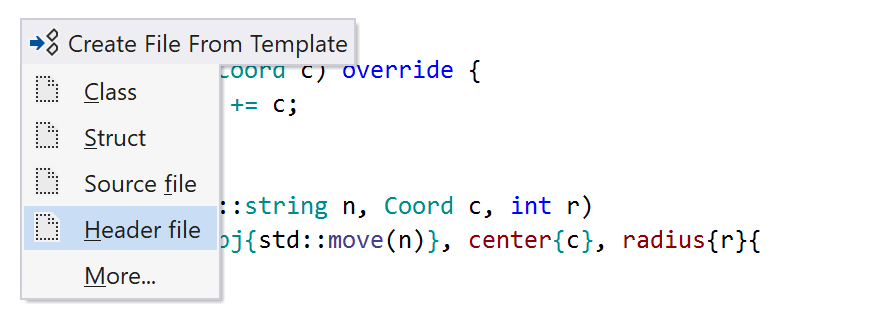
Postfix Templates
Postfix templates help you transform expressions that you have already typed without jumping backwards — just type a dot after an expression and pick a template from the completion list.
Below is the list of postfix templates available in C++.
Shortcut | Description | Example |
---|---|---|
.beg..end | Produces iterators from range |
|
.Cast | Surrounds expression with UE cast |
|
.cbeg..cend | Produces iterators from range |
|
.co_await | Passes expression as argument to co_await |
|
.co_return | Returns expression from current coroutine |
|
.co_yield | Passes expression as argument to co_yield |
|
.const_cast | Surrounds expression with const_cast |
|
.do | Iterating until boolean expression becomes 'false' |
|
.dynamic_cast | Surrounds expression with dynamic_cast |
|
.else | Checks boolean expression to be 'false' |
|
.foreach | Iterates over range |
|
.forward | Forwards function parameter |
|
.if | Checks boolean expression to be 'true' |
|
.make_shared | Constructs an object and wraps it in a std::shared_ptr |
|
.make_unique | Constructs an object and wraps it in a std::unique_ptr |
|
.new | Produces instantiation expression for type |
|
.reinterpret_cast | Surrounds expression with reinterpret_cast |
|
.return | Returns expression from current function |
|
.safe_cast | Surrounds expression with safe_cast (C++/CLI) |
|
.static_cast | Surrounds expression with static_cast |
|
.switch | Produces switch over integral/enum type |
|
.var | Introduces variable for expression |
|
.while | Iterating while boolean expression is 'true' |
|