String, Date, and Period Operations
The YouTrack workflow language supports several string and date operations that are used in standard Java libraries.
String Operations | ||||||
---|---|---|---|---|---|---|
Period Operations | ||
---|---|---|
The syntax for these operations is applied differently from other programming languages. When you want to use an operation, simply write the operation after the method or property that returns the appropriate data type. The syntax for each operation is presented in the format: [name]([data type] parameter): [data type]
.
The workflow editor applies constraints that let you enter only parameters and options that are supported by the workflow programming language. With code completion, the workflow editor displays a description and the available parameters for each operation.
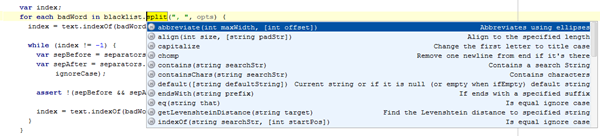
The following example demonstrates how to use an operation in a workflow rule.
var extractedUsername = comments.added.first.text.substringBetween("@", " ");
project.getUser(extractedUsername).notify("[Youtrack, Comment notice]", "You were mentioned in the comment of issue " + "", true);
This rule extracts the login for a user who is mentioned in comment from the @username
substring and sends a notification to the mentioned user.
String Operations
The following operations can be used with fields, methods, and operations that return a string
data type.
isEmpty(): Boolean | |
---|---|
Description | Checks whether the preceding string is empty ("") or null. |
isNotEmpty(): Boolean | |
---|---|
Description | Checks whether the preceding string is not empty ("") and not null. |
isBlank(): Boolean | |
---|---|
Description | Checks whether the preceding string is whitespace, empty ("") or null. |
isNotBlank(): Boolean | |
---|---|
Description | Checks whether the preceding string is not whitespace, not empty ("") and not null. |
trim(enum side[both, start, end], enum mode[asis, blankToNull, nullToEmpty]): string | ||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | side | Determines which side of the string is trimmed. | ||||||||||||||||||
mode | Determines how to handle blank and null values. | |||||||||||||||||||
Description | Removes whitespace from the beginning, end, or both sides of a string. The parameters determine which of the following operations is performed. | |||||||||||||||||||
Operations |
|
eq(string that, flag ignoreCase): Boolean | |||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | that | The string to compare to the preceding string. | |||||||||
ignoreCase | Determines whether the comparison is case sensitive. | ||||||||||
Description | Checks whether a string matches a specified string. The parameters determine which of the following operations is performed. | ||||||||||
Operations |
|
indexOf(string searchStr, [int startPos], flag last): integer | |||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | searchStr | The string to find in the preceding string. | |||||||||||||||
startPos | Sets the starting position inside the string. | ||||||||||||||||
last | Determines whether to search from the start or end of the string. | ||||||||||||||||
Description | Finds an index within a string. The parameters determine which of the following operations is performed. | ||||||||||||||||
Operations |
|
ordinalIndexOf(string searchStr, int ordinal): integer | ||
---|---|---|
Parameters | searchStr | The string to find in the preceding string. |
ordinal | The n-th | |
Description | Finds an ordinal index within a string. |
contains(string searchStr, flag ignoreCase): Boolean | |||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | searchStr | The search string to find in the preceding string. | |||||||||
ignoreCase | Determines whether the comparison is case sensitive. | ||||||||||
Description | Checks if a string contains a search string. The parameters determine which of the following operations is performed. | ||||||||||
Operations |
|
containsChars(string searchStr, enum mode[any, none, only]): Boolean | ||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | searchStr | The search characters to find in the preceding string. | ||||||||||||
mode | Determines whether to find any, none, or only the specified characters. | |||||||||||||
Description | Checks if a string contains a search character. The parameters determine which of the following operations is performed. | |||||||||||||
Operations |
|
substring(int start, [int end]): string | |||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | start | The position to start from. A negative integer counts back from the end of the string. | |||||||||
end | The position to end at (exclusive). A negative integer counts back from the end of the string. | ||||||||||
Description | Gets a substring from the specified string avoiding exceptions. The parameters determine which of the following operations is performed. | ||||||||||
Operations |
|
substringOfLength(int length, [int fromPosition], enum side[left, mid, right]): string | ||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | length | The length of the required string. | ||||||||||||
fromPosition | The position to start from. A negative integer is treated as zero. | |||||||||||||
side | Determines whether the string is taken from the left, middle, or right side of the string. | |||||||||||||
Description | Returns a substring of a specified length from the preceding string. The parameters determine which of the following operations is performed. | |||||||||||||
Operations |
|
substringRelative(string separator, enum pos[before, after], flag last): string | |||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | separator | The string to search for. | |||||||||||||||
pos | The position to start from. A negative integer is treated as zero. | ||||||||||||||||
last | Determines whether to search for the first or last occurrence of the separator. | ||||||||||||||||
Description | Checks whether a string contains a specified substring relative to a specified separator. The parameters determine which of the following operations is performed. | ||||||||||||||||
Operations |
|
substringBetween(string open, string close): string | ||
---|---|---|
Parameters | open | The string before the substring. |
close | The string after the substring. | |
Description | Returns a string that is nested in between two strings. Only the first match is returned. |
split([string separator], [int max], flag preserveAllTokens): sequence<string> | |||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | separator | The character to use as a delimiter. | |||||||||||||||||||||
max | The maximum number of elements to include in the sequence. A zero or negative value implies no limit. | ||||||||||||||||||||||
preserveAllTokens | Determines whether adjacent separators are treated as separators for empty tokens. | ||||||||||||||||||||||
Description | Splits a string into a sequence. The parameters determine which of the following operations is performed. | ||||||||||||||||||||||
Operations |
|
remove(string remove, flag ignoreCase, enum side[start, end]): string | |||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | remove | The string to remove from the preceding string. | |||||||||||||||
ignoreCase | Determines whether the comparison is case sensitive. | ||||||||||||||||
side | Determines whether to search from the start or end of the preceding string. | ||||||||||||||||
Description | Removes all occurrences of a substring from within the source string. The parameters determine which of the following operations is performed. | ||||||||||||||||
Operations |
|
replace(string searchString, string replacement, [int max]): string | |||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | searchString | The string to find in the preceding string. | |||||||||
replacement | The string to replace the | ||||||||||
max | The maximum number of values to replace. | ||||||||||
Description | Replaces all occurrences of a string within another string. The parameters determine which of the following operations is performed. | ||||||||||
Operations |
|
replaceChars(string searchChars, string replaceChars): string | ||
---|---|---|
Parameters | searchChars | The characters to find in the preceding string. |
replaceChars | The characters to replace the | |
Description | Replaces multiple characters in a string in one go. This method can also be used to delete characters. |
chomp(): string | |
---|---|
Description | Removes a new line from the end of a string, if present. |
repeat(int times): string | ||
---|---|---|
Parameters | times | The number of times to repeat the preceding string. |
Description | Repeat a string |
align(int size, [string padStr], enum to[left, right, center]): string | |||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | size | Specified the length of the new string. | |||||||||||||||||||||
padStr | Determines which characters are added to the preceding string. | ||||||||||||||||||||||
to | Determines which part of the string the | ||||||||||||||||||||||
Description | Align a string to the specified length. The parameters determine which of the following operations is performed. | ||||||||||||||||||||||
Operations |
|
length(): integer | |
---|---|
Description | Gets a the length of a string or 0 if the string is null. |
upperCase(): string | |
---|---|
Description | Converts a string to upper case. |
lowerCase(): string | |
---|---|
Description | Converts a string to lower case. |
capitalize(): string | |
---|---|
Description | Capitalizes a string, changing the first letter to title case. |
uncapitalize(): string | |
---|---|
Description | Uncapitalizes a string, changing the first letter to lower case. No other letters are changed. |
is(flag alpha, flag space, flag numeric): Boolean | ||||||||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | alpha | Determines whether to search for alphabetic characters. | ||||||||||||||||||||||||
space | Determines whether to search for space characters. | |||||||||||||||||||||||||
numeric | Determines whether to search for numeric characters. | |||||||||||||||||||||||||
Description | Checks a string for specific characters. The parameters determine which of the following operations is performed. | |||||||||||||||||||||||||
Operations |
|
isAsciiPrintable(): Boolean | |
---|---|
Description | Checks if the string contains only ASCII printable characters. |
default([string defaultString], flag ifEmpty): string | ||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | defaultString | The default string to return if the input is null. | ||||||||||||
ifEmpty | Determines how to handle blank values. | |||||||||||||
Description | Returns either the current string, an empty string, or the value of a default string. The parameters determine which of the following operations is performed. | |||||||||||||
Operations |
|
abbreviate(int maxWidth, [int offset]): string | |||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | maxWidth | The maximum length of the result string. Must be a value of 4 or higher. | |||||||||
offset | The left edge of the source string. | ||||||||||
Description | Abbreviates a string using ellipses. The parameters determine which of the following operations is performed. | ||||||||||
Operations |
|
getLevenshteinDistance(string target): integer | ||
---|---|---|
Parameters | target | The string to compare to the preceding string. |
Description | Finds the Levenshtein distance between the preceding string and a target string. |
startsWith(string prefix, flag ignoreCase): Boolean | |||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | prefix | The string to find at the start of the preceding string. | |||||||||
ignoreCase | Determines whether the comparison is case sensitive. | ||||||||||
Description | Check if a string starts with a specified prefix. The parameters determine which of the following operations is performed. | ||||||||||
Operations |
|
endsWith(string suffix, flag ignoreCase): Boolean | |||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|
Parameters | suffix | The string to find at the end of the preceding string. | |||||||||
ignoreCase | Determines whether the comparison is case sensitive. | ||||||||||
Description | Check if a string ends with a specified suffix. The parameters determine which of the following operations is performed. | ||||||||||
Operations |
|
Date Operations
The following operation can be used with fields, methods, and operations that return a date
data type.
format(string format): string | ||
---|---|---|
Parameters | format | The format to apply to the preceding date value. |
Description | Applies the specified UTC format to the date value. Returns a string. You can select a pre-defined date format or enter a custom format. Custom date formats start with |
The difference between two fields with date data types is returned as a duration. The following operation can be used with an operation that returns a duration
data type.
millis(): long | |
---|---|
Description | Returns the the number of milliseconds in a duration as a long number. |
Period Operations
The following operations can be used with fields that return a period
data type.
getPeriodFieldValueInMinutes(string fieldName): integer | ||
---|---|---|
Parameters | fieldName | The name of a custom field that stores a period type. |
Description | Returns the value that is stored in the specified custom field. Returns the value in minutes as an integer. |
getPeriodFieldOldValueInMinutes(string fieldName): integer | ||
---|---|---|
Parameters | fieldName | The name of a custom field that stores a period type. |
Description | Returns the previous value that was stored in the specified custom field before the changes that are applied in the current transaction. Returns the value in minutes as an integer. |
setPeriodFieldValueInMinutes(string fieldName, int value): void | ||
---|---|---|
Parameters | fieldName | The name of a custom field that stores a period type. |
value | The value in minutes to assign to the specified custom field. | |
Description | Assigns a value to the specified custom field. |