Getting Started with PyCharm
Everything you need to know to get started developing applications in PyCharm.
This tutorial will take you through installing and setting up PyCharm, customising the user interface, configuring your Python interpreters, managing your Python packages, getting assistance writing your code, and then running, refactoring and debugging your code.
Sample code
This is the code that we will be using in this tutorial:
class Car:
def __init__(self, speed=0):
self.speed = speed
self.odometer = 0
self.time = 0
def say_state(self):
print("I'm going {} kph!".format(self.speed))
def accelerate(self):
self.speed += 5
def brake(self):
if self.speed < 5:
self.speed = 0
else:
self.speed -= 5
def step(self):
self.odometer += self.speed
self.time += 1
def average_speed(self):
if self.time != 0:
if self.time != 0:
return self.odometer / self.time
else:
pass
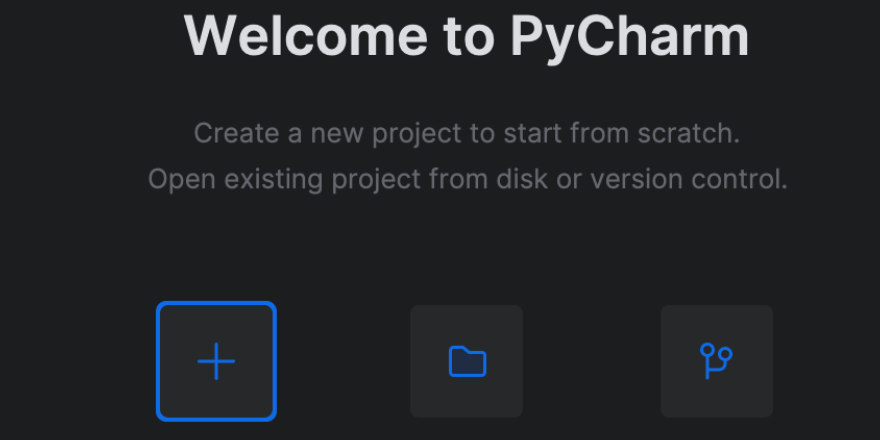
Installation and Setup
How to install and set up PyCharm.
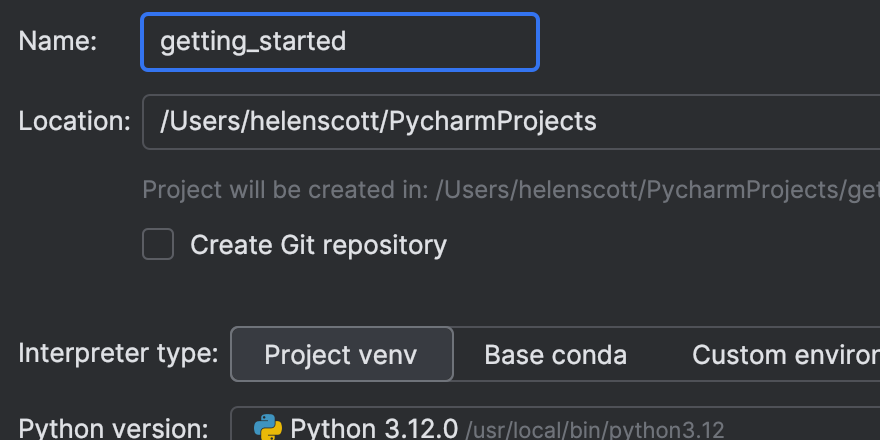
Your First Project
New to PyCharm? Start here!
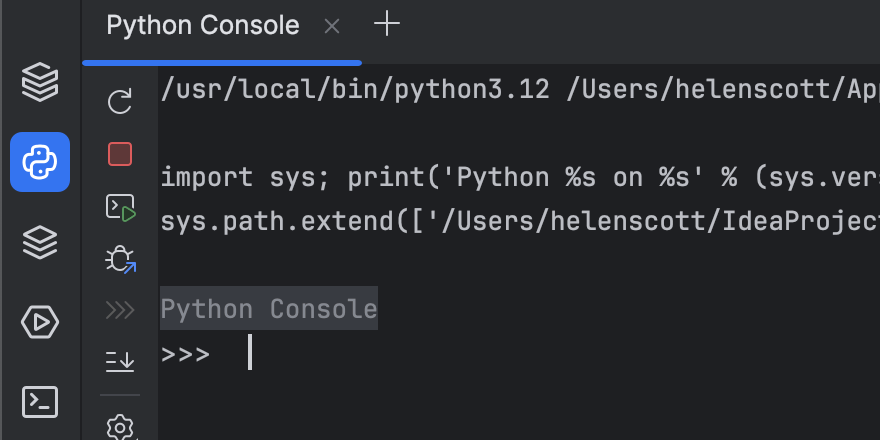
Understanding the UI
Finding your way around the user interface of PyCharm.
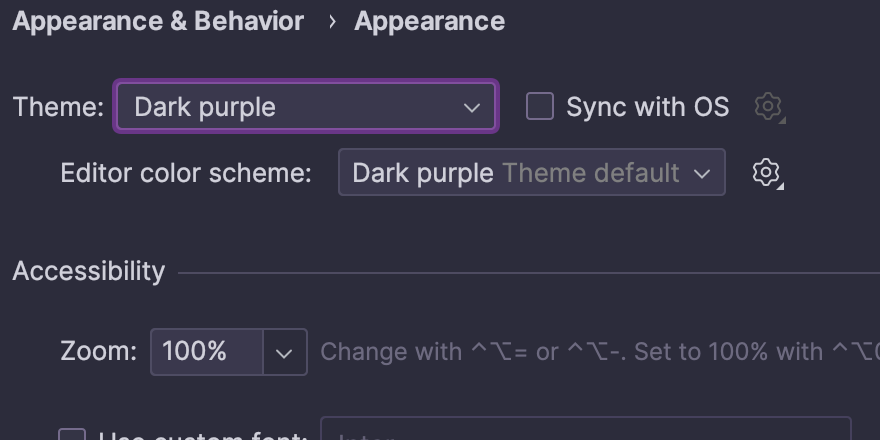
Customizing the UI
How to configure your UI exactly as you want it.
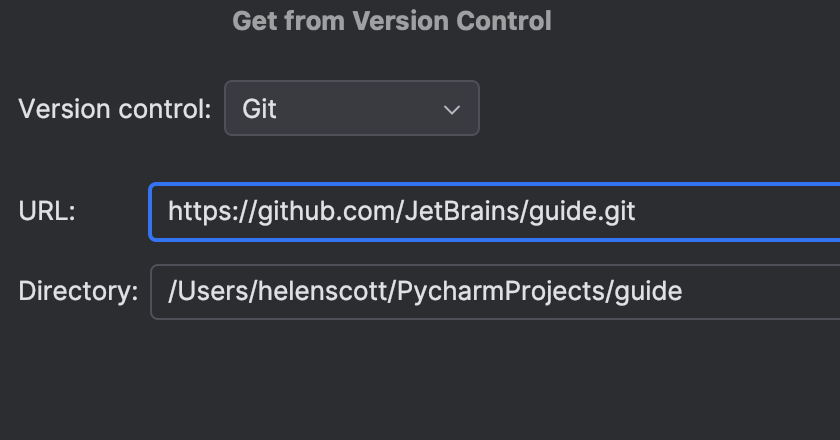
Configuring a Local Python Interpreter
How to set up a local Python interpreter for your project.
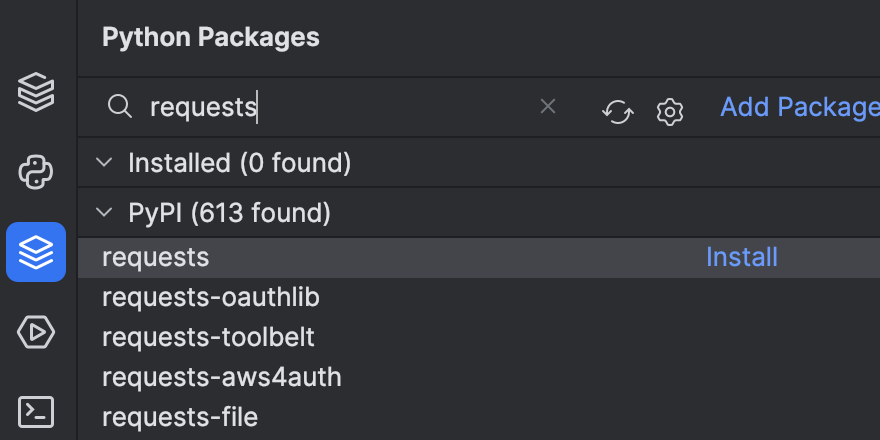
Installing and Managing Python Packages
How to work with Python packages in your project.
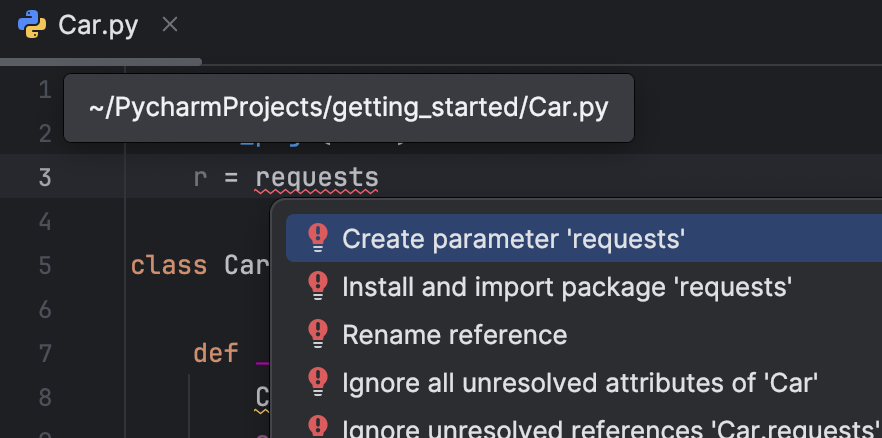
Basic Code Assistance
Learn about syntax highlighting and how to get code assistance.
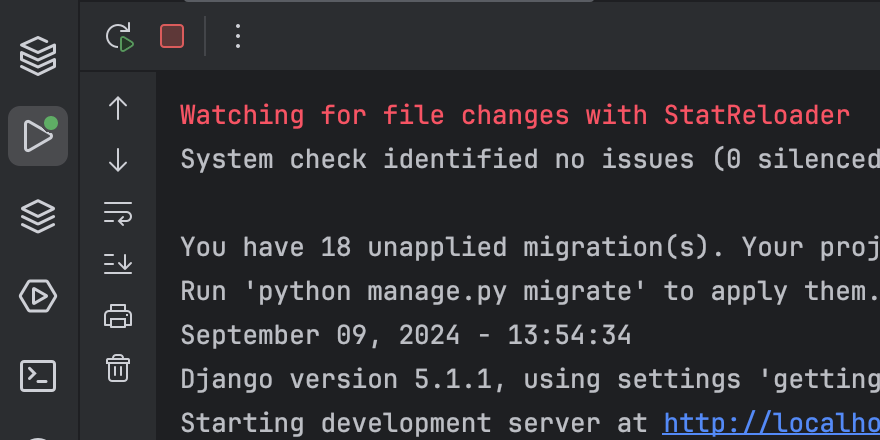
Run Python Using a Run Configuration
How to run you code in PyCharm.
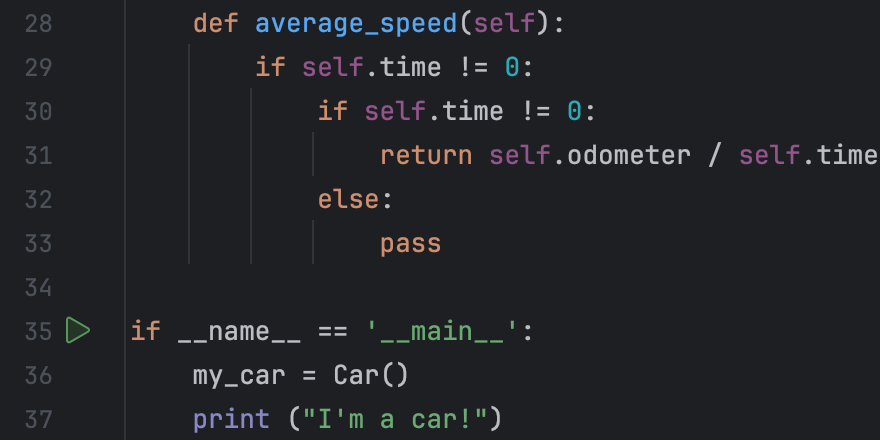
Basic Code Refactoring
Get more productive and less error-prone with refactorings.
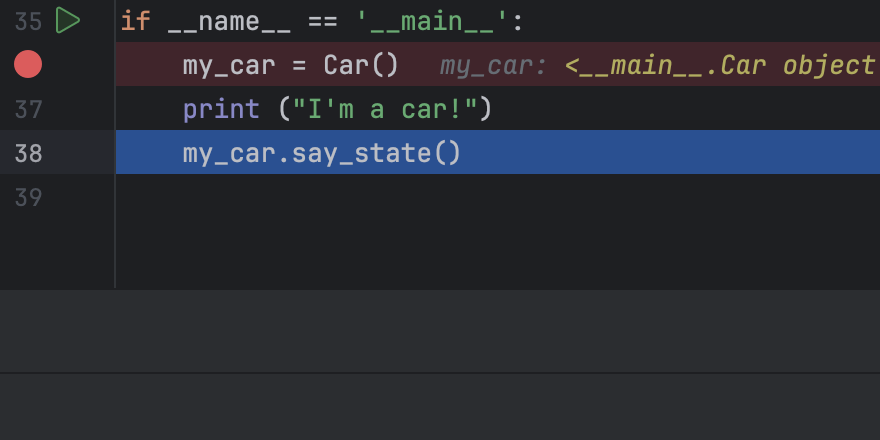
Basic Code Debugging
How to use PyCharm's debugger effectively.
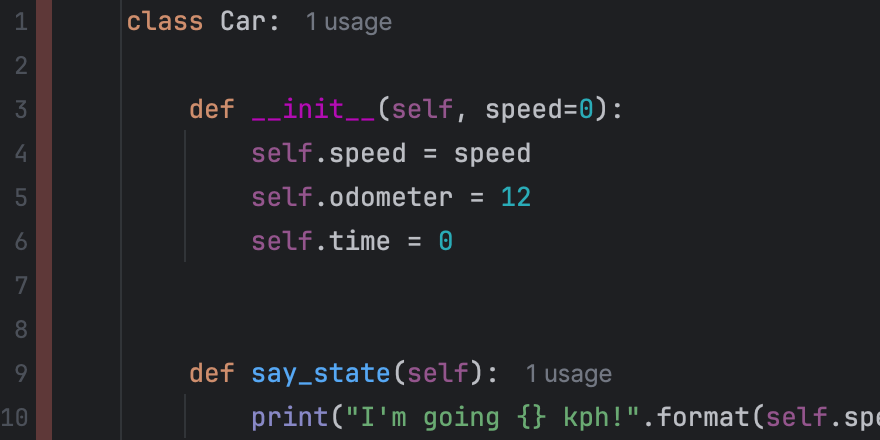
Basic Testing
Learn about syntax highlighting and how to get code assistance.
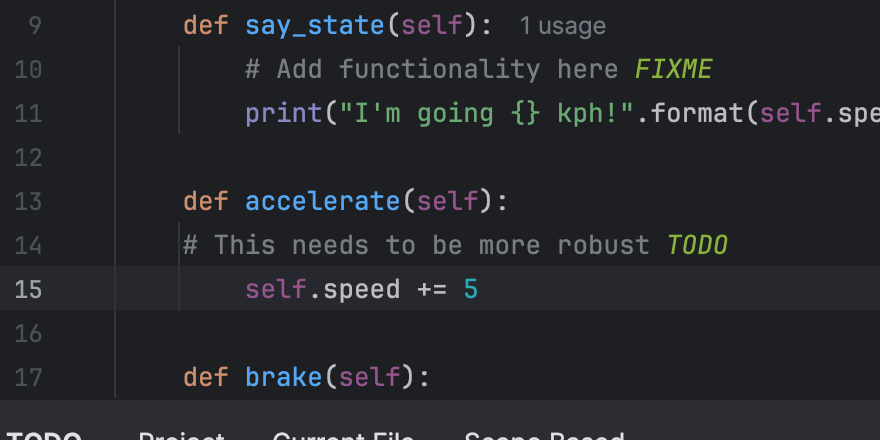
Productive Coding in PyCharm
Learn about syntax highlighting and how to get code assistance.