Building the Notes App with a database and a web form
This tutorial will guide you through creating a simple notes application using Go, SQLite, and a basic HTML/JavaScript form to add and delete notes.
Prerequisites
Go installed on your machine (version 1.16 or higher)
GoLand – most versions will do. This tutorial was created using GoLand 2024.2.
Internet connection to fetch the SQLite dependency.
Steps
Step 1: Initialize the project
Create a new directory for your project and initialize a Go module.
Click
.Alternatively, on the Welcome to GoLand screen, click New Project.
In the New Project dialog, select Go.
In the Location field, type or navigate to the location where you want to store your project.
In the GOROOT field, specify the location of your Go installation. GoLand typically detects this location automatically.
To change or install a new version of the Go SDK, click Add SDK (
) and select one of the following options:
Local: to select a Go SDK version on your local drive.
Download: to download the Go SDK from the official repository.
Clear the Add sample code checkbox. You can leave this checkbox, if you want to have some onboarding tips before you start.
Click Create.
GoLand creates a project folder and initializes a project, performing the following Bash actions instead of you:
mkdir notes-app cd notes-app go mod init notes-app
Step 2: Set up the database
Now let's create a new file named database.go. In this file, we will set up the SQLite database connection. The overall configuration includes the creation of the database file (notes.db) and the notes table, in which we will store information.
In the root directory of your project, create database.go with the following content:
The following procedure will guide you through the process of file creation.
Create a Go file
A new project has no Go files. To create a Go file, perform one of the following actions:
In the Project tool window, right-click the parent folder of a project, and select .
Click the parent folder of a project, press Alt+Insert, and select Go File.
Click the parent folder of a project, navigate to
.
In the New Go File dialog, type a name of the file and select whether you want to create an empty Go file (Empty file) or a Go file with the defined
main
function (Simple application).
Step 3: Create the Note struct
Create a new file named note.go
and define the Note
struct.
The Note
struct defines the structure of a note, which includes an ID, title, and content. This struct provides a clear and organized way to represent a note in the application.
Also, when interacting with the database, it's important to have a structured way to handle the data. The Note
struct allows you to map database rows to Go objects and vice versa. For example, when retrieving notes from the database, you can scan each row into a Note
struct.
Step 4: Implement CRUD operations
Let's add CRUD operations in note.go
. CRUD operations are the basic functions that are necessary to interact with a database or persistent storage. CRUD stands for Create, Read, Update, and Delete.
Create
operations are used to add new records or data entries to the database. In SQL, this is typically done using the INSERT statement.
Read
operations are used to retrieve existing records or data from the database. In SQL, this is typically done using the SELECT statement.
Update
operations are used to modify existing records or data in the database. In SQL, this is typically done using the UPDATE statement.
Delete
operations are used to remove existing records or data from the database. In SQL, this is typically done using the DELETE statement.
Step 5: Create the main function
Now let's modify main.go
and add the main
function there. Code in main.go
will set up a basic web server with routes to display notes, add new notes, and delete existing notes. It uses a SQLite database to store the notes and handles HTTP requests to perform CRUD operations.
To handle HTTP requests, we will use handlers. HTTP handlers in Go are functions that handle HTTP requests. They are the building blocks for creating web servers in Go. An HTTP handler takes an HTTP request, processes it, and generates an HTTP response. In Go, these handlers are functions or methods that satisfy the http.Handler
interface or are passed directly to functions like http.HandleFunc
.
We will start with the main
function.
Function: main
The main
function will perform the following functionality:
Database initialization:
initDB()
is called to set up the database connection. Thedefer db.Close()
statement ensures that the database connection is closed when the program exits.HTTP handlers: the
http.HandleFunc
function sets up routes and their corresponding handler functions:/
is handled byindexHandler
./add
is handled byaddHandler
./delete
is handled by deleteHandler.
When we run our program, the code will start Starting the Server: The server is started on port 8080, and any errors are logged and terminate the program.
Function: the index handler
The index handler performs several functions:
Retrieve notes: calls
GetNotes()
to get all notes from the database. If there is an error, it sends an internal server error response.Parse template: parses the
index.html
template file. If there is an error parsing the template, it sends an internal server error response.Execute template: executes the template with the retrieved notes, rendering the HTML to the response writer
w
.
Function: the add handler
The add
handler ensures that the note will be added to the database. The handler includes the following functionality:
Check request method: ensures that only POST requests are handled.
Retrieve form values: gets the title and content values from the form.
Create note: calls CreateNote to add a new note to the database.
Redirect: redirects the user back to the homepage after adding the note.
Function: the delete handler
The delete handler deletes notes from the database. This handler does the following:
Convert ID: Converts the id form value to an integer. If the conversion fails, it sends a bad request response.
Delete note: Calls DeleteNote to remove the note with the given ID from the database.
Redirect: Redirects the user back to the homepage after deleting the note.
Step 6: Create the HTML form
Now let's create a new file named index.html
with a form to add and delete notes. This file will use html/template
package. The html/template
package in Go provides the functionality to parse and execute templates. The placeholders like {{range .}}
and {{.Title}}
are part of Go's template syntax, allowing dynamic content to be inserted into the HTML.
Here is some breakdown of the syntax used in our HTML template:
{{range .}}
: starts a loop over a collection of data passed to the template. In this case,.
represents the data passed to the template, which is a slice ofNote
structs.{{range .}}
iterates over each element in the collection.{{.Title}}
: inside the{{range .}}
block,.
refers to the current element of the iteration.{{.Title}}
accesses theTitle
field of the currentNote
struct.{{else}}
: provides an alternative content to display if the range does not iterate over any elements (for example, if the collection is empty).{{end}}: marks the end of the range block.
Connection with the Go code
In the indexHandler function in your Go code, you retrieve the notes from the database and pass them to the template.
In the following code snippet, template.ParseFiles("index.html")
reads and parses the index.html
file.
tmpl.Execute(w, notes)
executes the template, passing notes
as the data. This notes
slice of Note
structs is what {{range .}}
iterates over.
Step 7: Run the application
GoLand has various options to run your application. The easiest one is just right from editor.
Run from the editor
If you are not going to pass any parameters to your program, and your program does not require any specific actions to be performed before start, you can run it right from the editor.
Click
in the gutter near the
main
function and select Run.To run a script, open it in the editor or select it in the Project tool window, and then select Run <script file name> from the context menu.
Behind the scenes, GoLand runs go run main.go
amd displays the output in the Run tool window.
Now, you just need to open your web browser and navigate to http://localhost:8080. You should see a form to add notes and a list of existing notes with options to delete them.
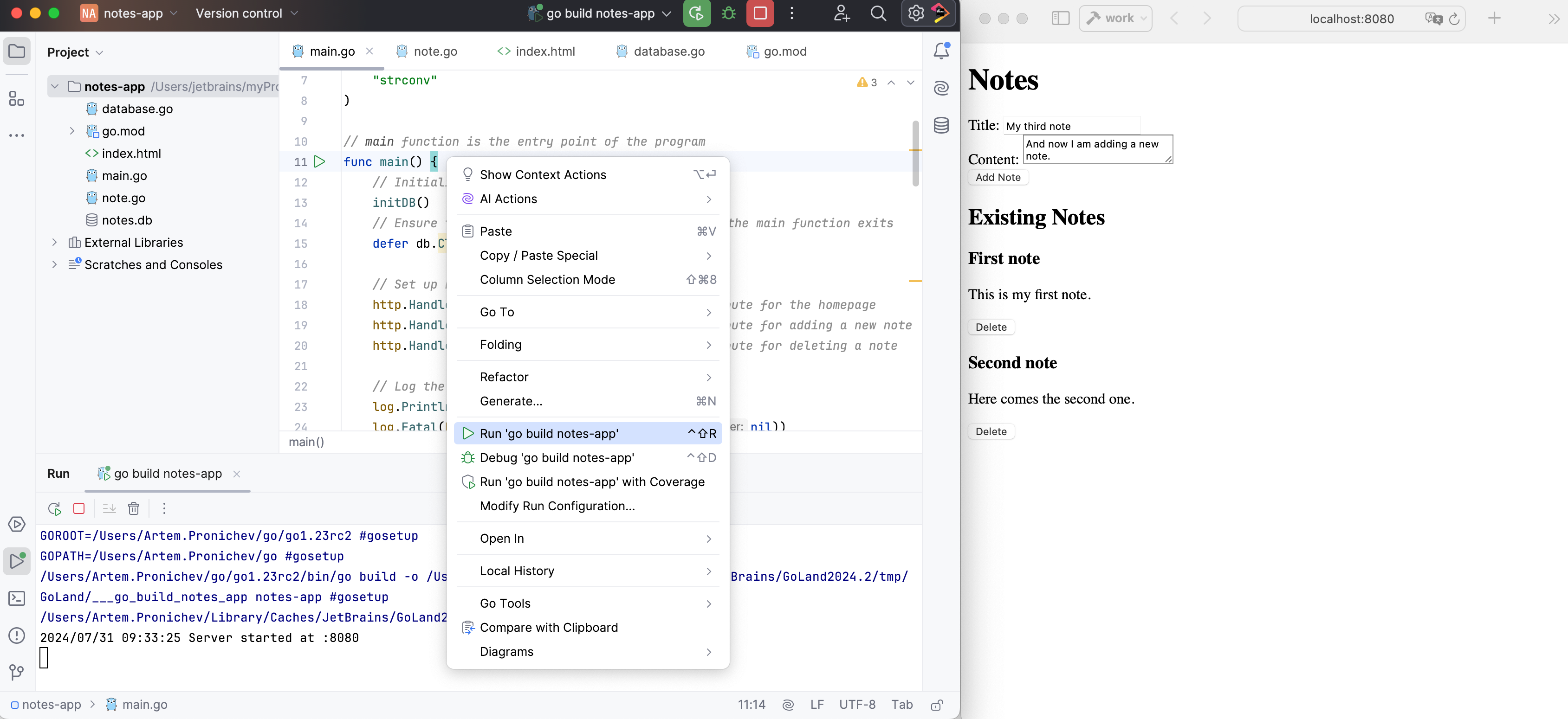