Tutorial: Build UI using Swing
The UI Designer plugin in IntelliJ IDEA enables you to create graphical user interfaces (GUI) for your applications using the Swing library components. Using UI Designer, you can quickly create dialogs and groups of controls to be used in top-level containers, such as JFrame. These elements can coexist with the components that you define directly in your Java code.
In this tutorial, you will learn the basics of working with UI Designer and try creating your own GUI form for a sample application. As an exercise, we will build a GUI form for editing the information about a book, such as its title, author, genre, and availability status.
At the end of the tutorial, your form will look similar to the following example:
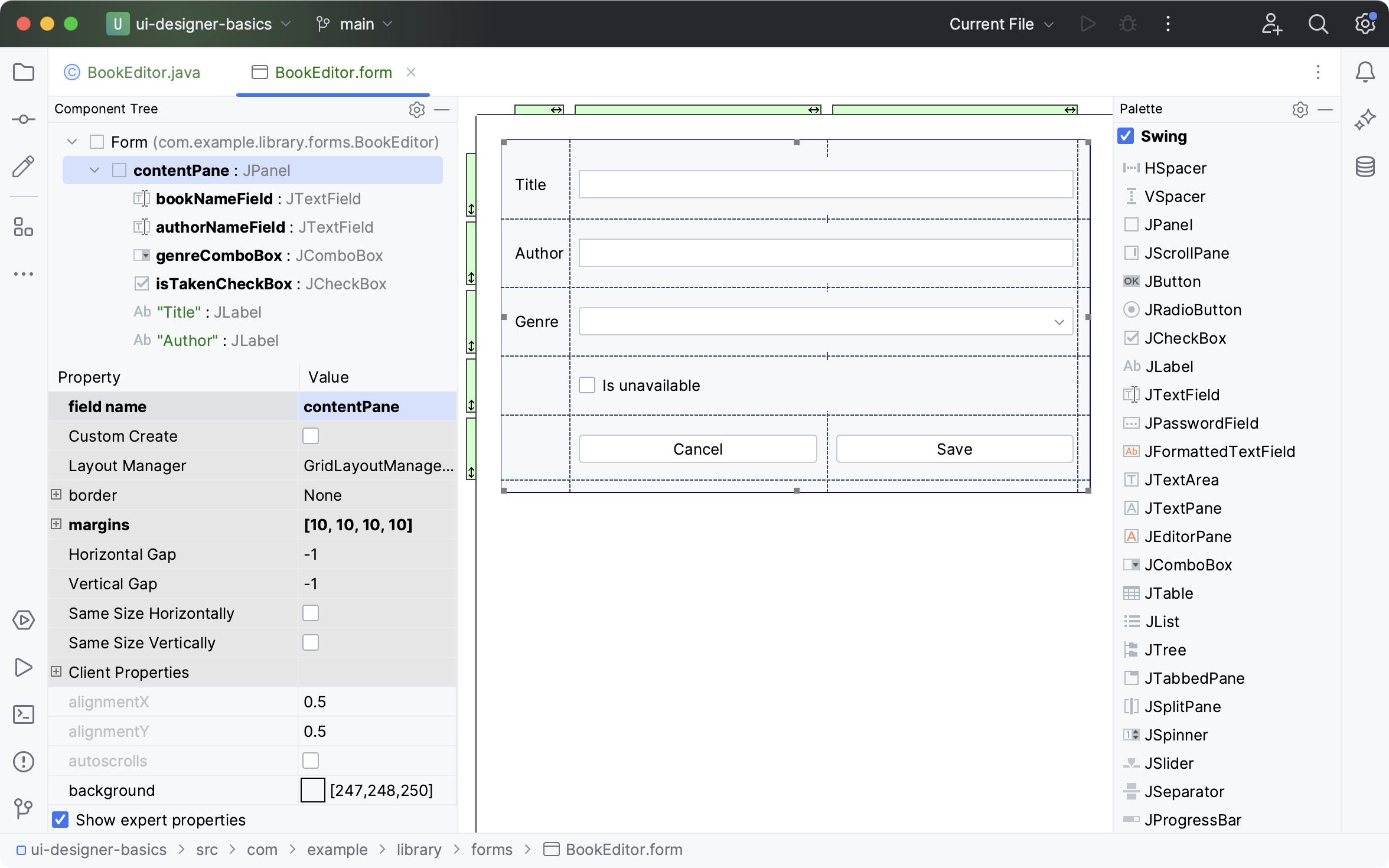
Install the Swing UI Designer plugin
This functionality relies on the Swing UI Designer plugin, which you need to install and enable.
Press Ctrl+Alt+S to open settings and then select
.Open the Marketplace tab, find the Swing UI Designer plugin, and click Install (restart the IDE if prompted).
Clone the sample project
The source code of the application is hosted on GitHub at Build UI using Swing Sample Project
In the main menu, go to
.Specify the URL of the repository and click Clone.
If necessary, agree to open the cloned project in a new window.
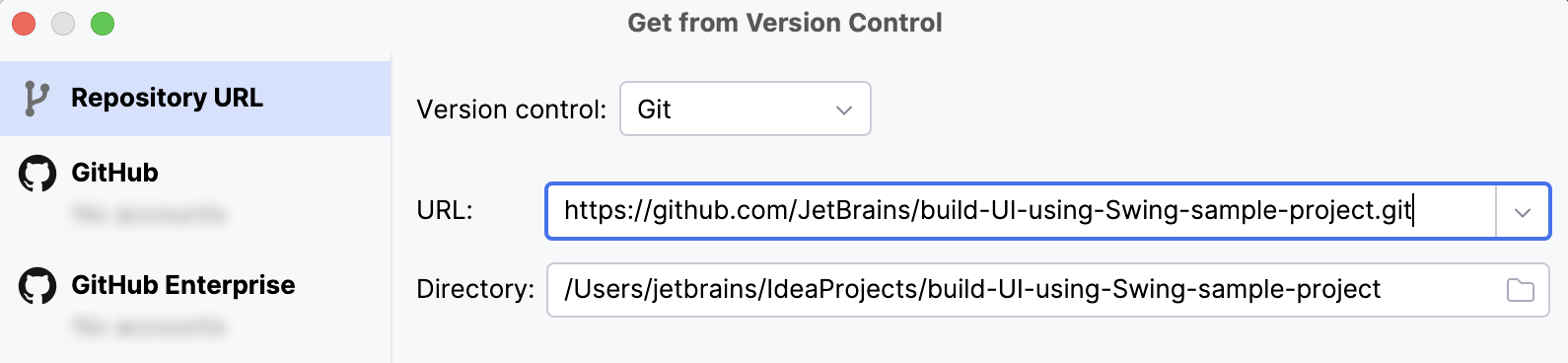
IntelliJ IDEA stores the information about GUI forms that you create in .form files. A form can be associated with a Java source file and put under version control.
As a first step, we will create a new GUI form and an associated Java class for it.
Create a new GUI form
In the Project tool window Alt+1, select the src.com.example.library.forms package, press Alt+Insert, and choose .
In the New GUI Form dialog that opens, specify the following details:
Form name: specify
BookEditor
as a name for the new GUI form.Base layout manager: select the
GridLayoutManager (IntelliJ)
layout manager from the dropdown.Create bound class: select the option to create an associated Java class together with the form.
IntelliJ IDEA will automatically create and bind a Java class to the GUI form. The bound class serves as the underlying code representation for a GUI form. It allows you to link components to corresponding fields in the class and facilitates the implementation of behavior and functionality for the form.
Class name: leave the default name for the Java class associated with the form.
Click OK.
Our project now has a BookEditor.form file and BookEditor.java file. The BookEditor.form file is where we will arrange the GUI components and set their properties. The BookEditor.java file will contain the code that will make our form functional.
Sections of a form
Before we proceed, let us take a quick look at BookEditor.form. When you select a .form file, you will have a view of the UI Designer which consists of four main sections.
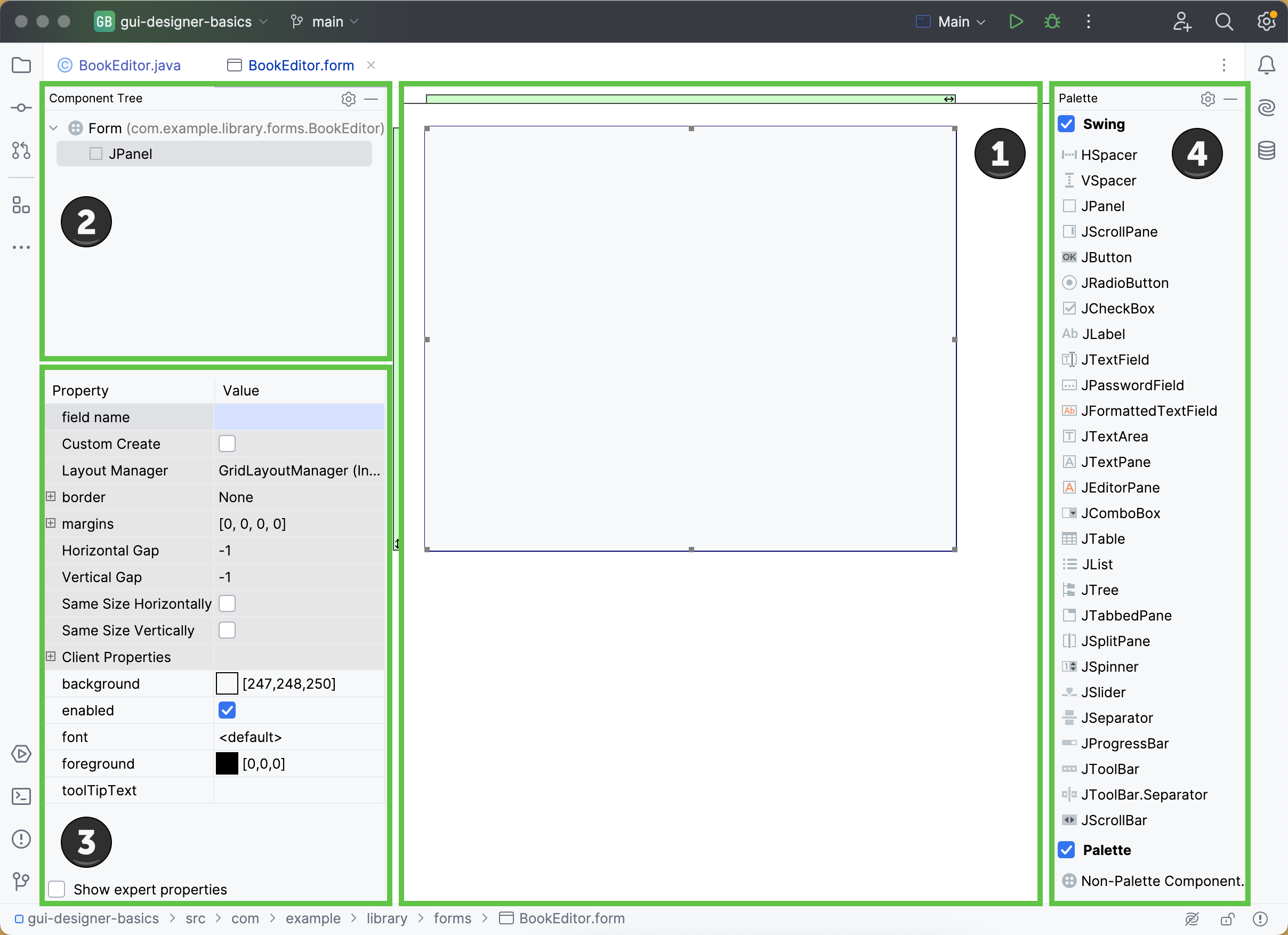
Form workspace: shows a visual representation of the components in your form. The top-level JPanel component is added by default when you create a new GUI form in IntelliJ IDEA, and you can add more components to build out your form.
Component Tree: shows the components contained in the design form and enables you to navigate and select one or more components. Our form currently only has one component, JPanel, however we will soon add more components.
Property inspector: shows properties for the component currently selected in the form workspace or the Component Tree.
Palette: contains a list of UI components you can place in a form.
Next, let us continue by building out our form and incorporating the required fields, checkboxes, and controls into our Book editor.
First, we can work on the JPanel component which acts as a container to store the GUI you want to build.
Set up the JPanel container
Open BookEditor.java and paste the following code at the top of the file under the package declaration:
import javax.swing.*;In BookEditor.java also paste the following code inside the
BookEditor
class:private JPanel contentPane;Open the BookEditor.form file.
In the Component Tree section, select JPanel to see the properties available for the component.
In the property inspector, set the value of the field name property to contentPane.
Place GUI components on the form
Open the BookEditor.form file.
In the Palette section, select JTextField and click the top section of the form to place the component.
Repeat the action and add one more JTextField, one JComboBox, and one JCheckBox component in this order from top to bottom.
IntelliJ IDEA displays the added components in the Component Tree and adds corresponding declarations to BookEditor.java.
Open BookEditor.java. Replace the
BookEditor
class with the content below in which we have renamed the field names and extedned theBookEditor
class:public class BookEditor extends JFrame { private JPanel contentPane; private JTextField bookNameField; private JTextField authorNameField; private JComboBox genreComboBox; private JCheckBox isTakenCheckBox; }Switch back to BookEditor.form. You will see that after changing the field names in BookEditor.java, IntelliJ IDEA displays errors in the Component Tree. To resolve these issues, set the updated component names as values of the field name property in the property inspector.
Our field names currently do not have any labels and are unrecognizable to end users. The Swing library provides a specific component, JLabel, that you can use to add any text labels to your GUI form.
For the checkbox, adding a label is not required, since you can configure the text directly in the JCheckBox component properties.
Add text labels
Add the JLabel component to the right of each JTextField and JComboBox.
Select the first JLabel in the Component Tree. In the property inspector, change the value of the text property to
Title
.Change the names of the other two labels to
Author
andGenre
in a similar manner.Select the JCheckBox component in the Component Tree. Then, specify
Is unavailable
as a value of the text property.
We want our Book editor to have the ability to save and discard the changes made by the end user. For this purpose, let us add the Save and Cancel buttons.
Add buttons
Add the VSpacer component below the cell with the JCheckBox. This will create an area for the buttons and divide it equally in two halves.
Add two JButton components to each side of the vertical spacer.
In the text property of each button, specify their names accordingly –
Cancel
andSave
.
Now that we have all the necessary Swing components placed to the GUI form, let us check the intermediate results and learn how the form will look at runtime.
Preview the GUI form
Right-click your GUI form and select Preview from the context menu.
IntelliJ IDEA will display the Form Preview dialog. While in Preview mode, you can click buttons, select checkboxes, enter text, and so on, just like you do at runtime.
At this stage, our GUI form is a little raw. Let us improve the look and feel of it by adjusting the margins and setting up the preferred window size.
Adjust the look and feel of the GUI form
Select the JPanel container in the Component Tree.
Use the margins group of properties to configure top, bottom, left, and right margins of the container. Set the value of each margin to
10
.To configure the preferred size of the form, select the Show expert properties checkbox, and then find and unfold the preferredSize group of properties. Set the value of the width property to
400
, and height to200
.Run preview one more time to check the results.
Now that the GUI form is ready, you can make it functional by editing the content in BookEditor.java. Functionality for the form in this tutorial has been prepared and is available in BookEditorExample.java which can be found under src/com/example/library/forms/BookEditorExample.
Add functionality to the form
Replace all the contents of BookEditor.java with the contents from BookEditorExample.java.
In BookEditor.java, rename the two instances of
BookEditorExample
toBookEditor
in the class declaration and the public method declaration.Open Main.java and run the application.
The details of a book that you enter in the form will be printed out in the terminal.