Support for desktop accessibility features
Compose Multiplatform builds on top of Jetpack Compose, making most accessibility features available in common code across all platforms. The current status of accessibility support on desktop is as follows:
Platform | Accessibility status |
---|---|
MacOS | Fully supported |
Windows | Supported via Java Access Bridge |
Linux | Not supported |
Enabling accessibility on Windows
Accessibility on Windows is provided via Java Access Bridge, which is disabled by default. To develop accessibility features on Windows, enable Java Access Bridge with the following command:
To create a native distribution that includes accessibility features, add the jdk.accessibility
module using the modules
DSL method:
Example: Custom button with semantic rules
Let's create a simple app with a custom button and specify explanatory text for screen reader tools. With a screen reader enabled, you will hear the "Click to increment value" text from the button description:
To test accessibility information for the elements in your application on macOS, you can use Accessibility Inspector (Xcode | Open Developer Tool | Accessibility Inspector):
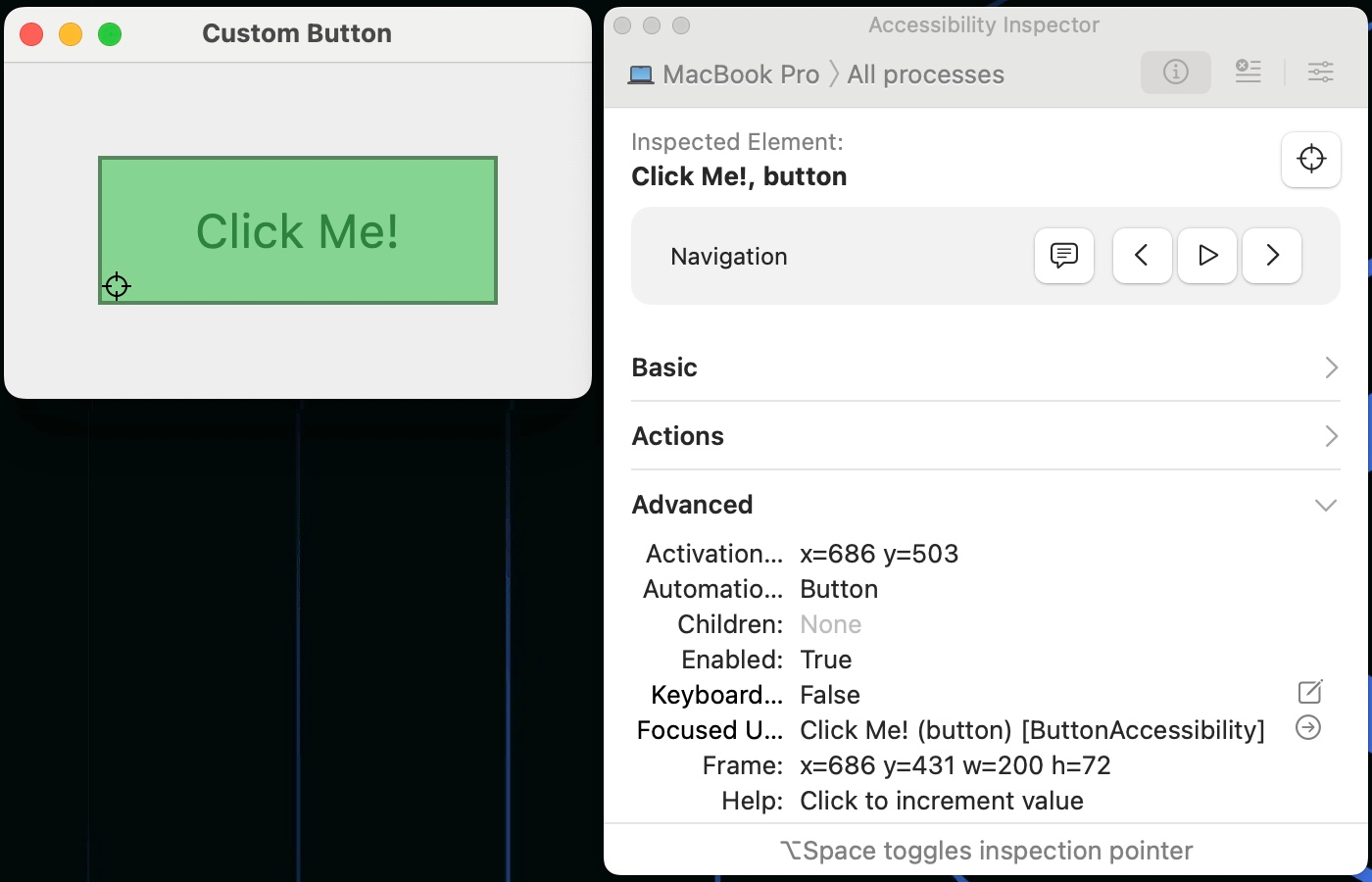
On Windows, you can use the Show Speech History feature in JAWS or the Speech Viewer in NVDA:
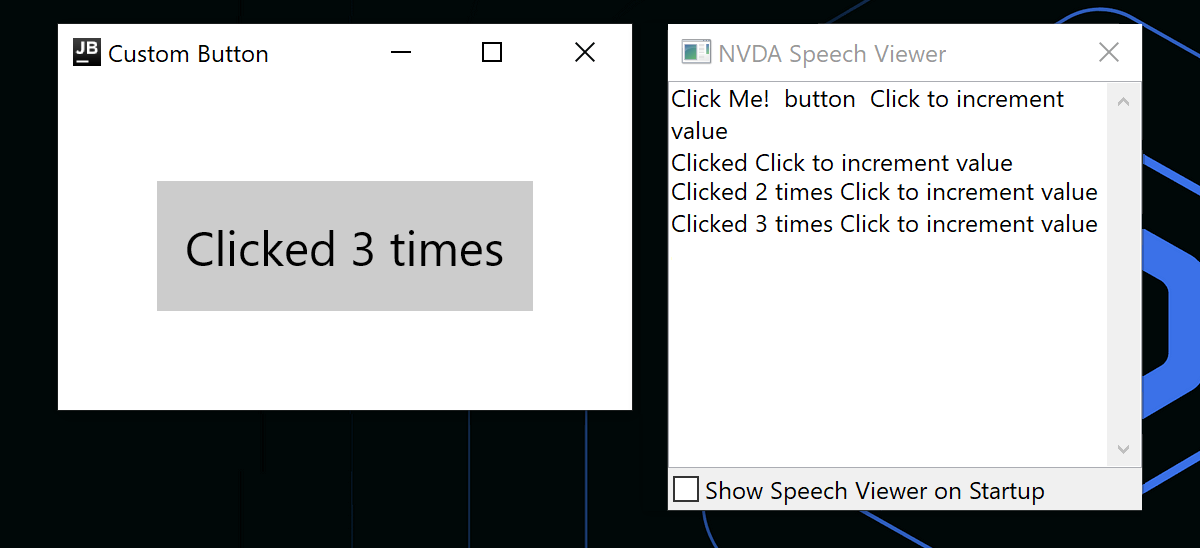
For more examples, refer to the Accessibility in Jetpack Compose guide.
What's next?
Explore the tutorials about other desktop components.