Debug .NET exceptions
tip
When copying an exception stack trace (for example, from an ASP.NET application) to the clipboard, JetBrains Rider allows navigating from that stack trace to the exception location.
An exception is an error that occurs in runtime and typically disrupts the normal execution flow of a program. An unhandled exception terminates the thread where it occurs. In a single-threaded app, this usually ends the entire process. In a multithreaded app, the process may continue if other threads are running.
To aid in ebugging, JetBrains Rider allows you to explicitly configure the debugger to suspend the program’s execution when any exception, or an exception of a specific type, is thrown. This suspension happens right after the exception occurs, before any exception handling is executed, enabling you to inspect the program's stack frames and the surrounding context.
To debug an exception, handled or unhandled, in your code or in the external code, you need to create a special exception breakpoint. Unlike line breakpoints, exception breakpoints are assigned not to a certain line of code, but to a certain exception type (for example, in case of .NET, it can be any type inherited from the Exception
type), or to any CLR exception.
Do one of the following:
Choose Run | Stop On Exception from the main menu.
In the Breakpoints dialog (CtrlAlt0B or Run | Debugger Actions | View Breakpoints...) , click
and select CLR Exception Breakpoints.
In the dialog that opens, specify the desired exception type name and click OK. The breakpoint is added to the list of breakpoints under CLR Exception Breakpoints in the Breakpoints dialog.
Specify breakpoint properties and click Done.
tip
You can add exception breakpoints both before starting a debugging session and during a debugging session.
The CLR Exception Breakpoints category in the Breakpoints dialog contains the Any exception breakpoint. When it is enabled, its properties will be used to match all .NET CLR exceptions thrown by your program.
When you add a breakpoint for a specific exception type to this category, it will override the properties of Any exception.
So you can enable the Any exception breakpoint, add a breakpoint for an exception type that you want to skip, and clear the Suspend execution checkbox in its properties. With this configuration, the debugger will stop on any exception excluding exceptions of the ignored type.
You can fine-tune this behavior even further, for example, you can select all check-boxes in the Suspend if group for the Any exception breakpoint and clear the External code checkbox for a specific exception type. This way, you will ignore only exceptions of the specific type that are thrown in the library code, and break at all other exceptions, if any.
note
All exception breakpoints that you want to apply, even as a way to ignore specific exception types, must have their Enabled checkbox selected.
Item | Description |
---|---|
Enabled | Select this checkbox to enable the selected breakpoint. If the breakpoint is disabled, it won't be hit during debugging. You can also use checkboxes in the left-hand-side view to enable/disable breakpoints. |
Suspend execution | Select this checkbox if you want the debugger to suspend the program when the breakpoint is hit. You can disable this option if you want the breakpoint only to log some data or calculate an expression at some point without suspending the program. |
Log | Select these checkboxes if you want to log the breakpoint hit in the debugger output. You can choose either to log the fact the breakpoint was hit or log the full stack trace of the program when the breakpoint was hit, or both. You would normally want to clear the Suspend checkbox when you use the breakpoint for logging. |
Disable until breakpoint is hit | From the list, select the breakpoint you want the current breakpoint to depend on. Besides that, you can also choose whether to disable the current breakpoint again after it is enabled and hit or to leave it enabled. |
Suspend if | This group of checkboxes allows you to configure whether to stop on tha exception depending on where it is thrown (User code or External code) and how it is handled. For example, you may want to stop the debugger only on exceptions that were thrown in the external code and handled in the user code. |
When an exception breakpoint is added, JetBrains Rider will break program execution when a matching exception is thrown and display the exception popup, which contains the exception type, its call stack with clickable links to the corresponding calls:
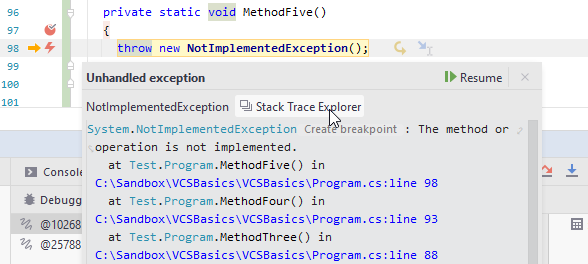
Use this popup to investigate the exception and the surrounding context:
Stop: stop the debugging session. This can be helpful if you break on a handled exception and do not want to go to the
catch
clause.Mute and Resume: resume program execution and add a new exception breakpoint that will act as a way to ignore similar breakpoints. For example, if you break on an unhandled
NotImplementedException
, a new exception breakpoint forNotImplementedException
will be created with the unselected Unhandled checkbox.Resume: resuming a program makes sense if the exception is handled, or if you are debugging a multithreaded process.
Stack Trace Explorer: open the exception details in the Stacktrace window where you can explore the stack trace right away or later.
View Breakpoint: open the properties of the exception breakpoint that was triggered by this exception.
Create breakpoint: create a new exception breakpoint that will match the exception type and context. For example, if the Any exception breakpoint was triggered by an unhandled
NotImplementedException
, a new breakpoint for unhandledNotImplementedException
wil be created.