Debugging with PhpStorm
Quick start
Below is a quick-start guide to using PhpStorm and Xdebug 3 for debugging PHP applications that run on a local or remote web server.
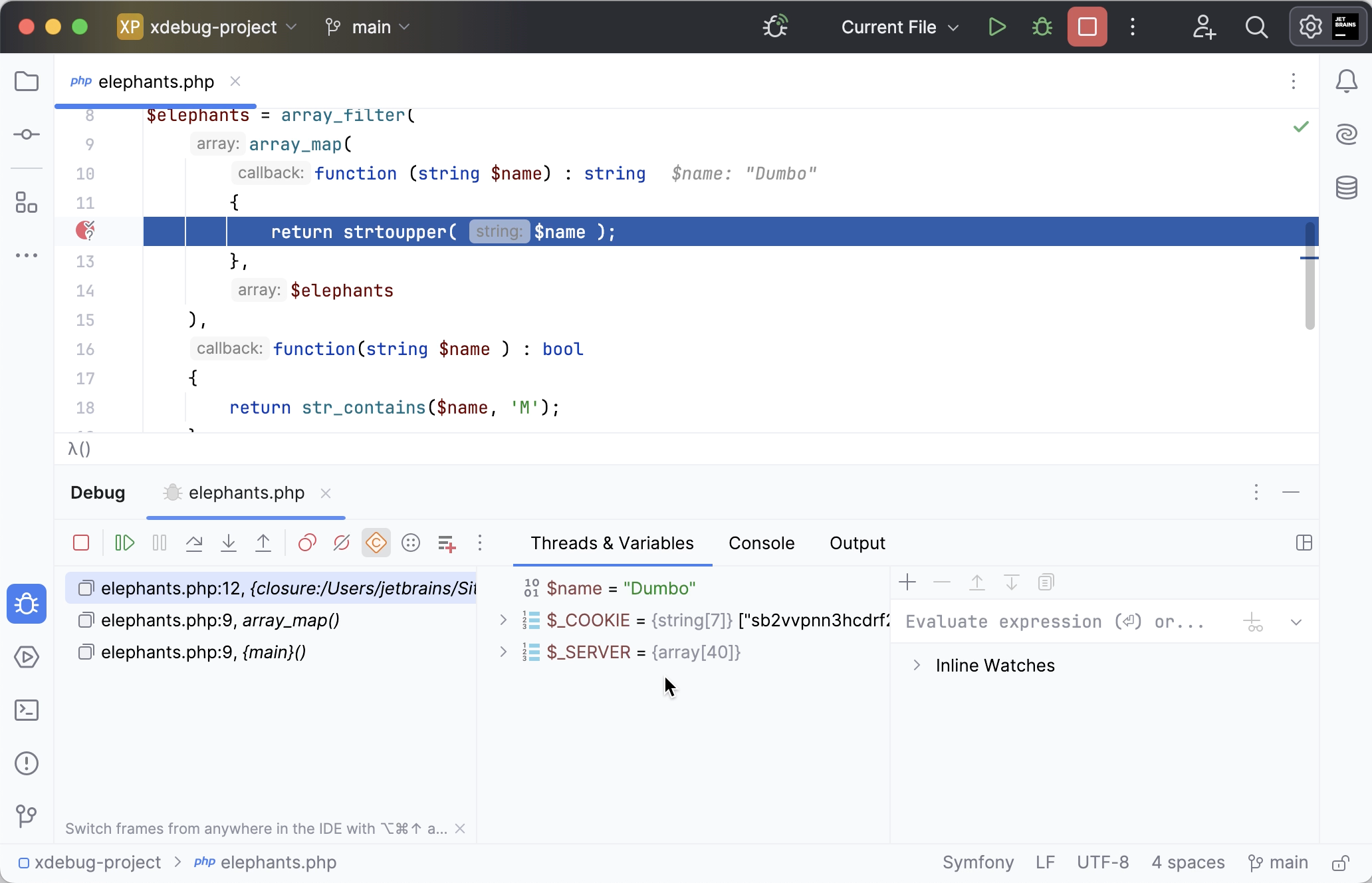
For other debugging scenarios such as debugging PHP tests or remote debugging via SSH, see Debugging scenarios.
1. Install Xdebug
The Xdebug debugging engine is an extension for PHP, so it is installed to PHP on the local or remote web server where your application runs. To install Xdebug:
Download the Xdebug extension compatible with your PHP version and install it as described in the Xdebug installation guide.
If you are using a preconfigured AMP (Apache, MySQL, PHP) package, the Xdebug extension may be already installed. Refer to the instructions specific for your package.
Edit the relevant php.ini file to integrate Xdebug with the PHP engine:
Disable the Zend Debugger and Zend Optimizer tools which block Xdebug. To do so, remove or comment out the following lines in the php.ini file:
zend_extension=<path_to_zend_debugger> zend_extension=<path_to_zend_optimizer>Enable Xdebug. To do so, locate or create the
[xdebug]
section in the php.ini file and update it as follows:[xdebug] zend_extension=xdebug xdebug.mode=debug xdebug.client_host=localhost xdebug.client_port=9003zend_extension
: the setting indicating that the Xdebug debugging engine shall be used. Set toxdebug
or use the full path to thexdebug.so
file, such as/usr/lib/php/20190902/xdebug.so
.xdebug.mode
: the setting controlling which Xdebug features are enabled. Set todebug
for debugging.xdebug.client_host
: the IP address or hostname at which PhpStorm is running and listening for incoming connections from Xdebug. For example,localhost
or127.0.0.1
if Xdebug and PhpStorm run on the same machine. Xdebug only supports connecting to a single IP address, however, you can use a DBGp proxy to debug PHP applications in multiuser environments.xdebug.client_port
: the port to which Xdebug tries to connect on the host where PhpStorm runs. The default port is9003
.
Verify Xdebug installation by doing any of the following:
In the command line, run the following command:
php --versionThe output should list Xdebug among the installed extensions:
Create a php file containing the following code:
<?php phpinfo();Open the file in the browser. The
phpinfo
output should contain the Xdebug section:Use PhpStorm (Validate the configuration of a debugging engine.
in the main menu) to validate the installation and configuration of Xdebug on your web server and get suggestions for fixing the inconsistencies if there are any. For details, see
2. Add a debugging extension to the web browser
The easiest way to instruct the web server to activate the debugger is to use a browser extension.
Choose and install the browser extension suitable for your browser — for example, Xdebug helper for Chrome.
For more options and details, see Browser debugging extensions.
Open the application URL in the browser and activate the browser extension.
3. Configure PhpStorm to listen to incoming connections
Check that the Debug port setting in corresponds to the port configured on the debbuger side. The defualt value is
9003
.Additionally, you can customize other Xdebug settings in PhpStorm as described in define Xdebug behaviour.
On the PhpStorm toolbar, toggle
to start listening for incoming PHP debug connections, or choose from the main menu.
4. Start a debugging session
Open the application development project in PhpStorm and set a breakpoint in code. Breakpoints can be set in the PHP context inside PHP, HTML, TWIG, BLADE, and files of other types.
Line breakpoints can be set by clicking the gutter at the executable line of code.
Conditional breakpoints are hit only when the specified condition, such as a specific item in the loop, is met. To specify a condition, set a line breakpoint and right-click it to open the breakpoint settings dialog.
With exception breakpoints, the debugger suspends execution of the application code when an exception or an error is thrown or when a PHP notice or warning is emitted. To set an exception breakpoint, see Debug with PHP exception breakpoints.
Alternatively, select
to have the debugger stop as soon as connection with PhpStorm is established (instead of running automatically until the first breakpoint is reached).Back in the browser, reload the application page to start the debugging session and return to PhpStorm.
As soon as PhpStorm detects a new incoming connection from Xdebug, it displays an Incoming Connection From Xdebug dialog.
In the Incoming Connection From Xdebug dialog, select the path mappings so that PhpStorm can map the remote files on the web server to the local files in your project. If you have a deployment configuration defined, PhpStorm will offer configuring the mappings based on the paths you've already set in that configuration.
5. Examine the program state
After reaching the breakpoint, the debugger is suspended. You can now investigate the application in PhpStorm's Debug tool window.
For more information about examining the program data (frames, variables, and so on), see Examine a suspended program.
Continue running the program and examine its frames as soon as it is suspended again.
To control the program execution manually, step through the code using the commands under the Run menu or toolbar buttons: F7, Shift+F8, F8, and others. For more information, refer to Step through the program.
To have the program run automatically up to the next breakpoint, resume the session by choosing
or pressing F9.
Debugging scenarios
The following topics will assist you in exploring debugging scenarios in PhpStorm:
- Zero-configuration debugging
The debugging scenario described in this Quick start guide uses the so-called zero-configuration debugging approach, which means that you do not need to create a run/debug configuration like you would for running or testing PHP applications in PhpStorm.
If you want to run debugging sessions using run/debug configurations, see Debug with a PHP web page debug configuration.
- Debugging a PHP CLI script
Besides running and debugging an entire application on the web server, you may want to debug a specific PHP script in PhpStorm, or run a PHPUnit, Behat, or other test with the debugging engine attached.
In this case, the debugging engine is set up together with the local or remote PHP interpreter configured in PhpStorm.
For more information, see Debug a PHP CLI script.
- Debugging PHP framework CLI commands
- Simultaneous debugging sessions
When building web applications with multiple tiers, you can have frontend PHP code calling into backend PHP code. Simultaneous debugging sessions describes several ways to step from front-end code into back-end code and debug them simultaneously.
- Multiuser debugging via Xdebug proxies
Xdebug only supports connecting to a single IP address at which your PhpStorm IDE is running. To debug PHP applications in multiuser environments, see Multiuser debugging via Xdebug proxies.
- Remote debugging via SSH tunnel
Remote debugging via SSH tunnel describes how to use an SSH tunnel to establish a secure connection between Xdebug and PhpStorm when they run on different machines, and direct connection between the two machines is not possible.
- Troubleshooting
See Troubleshooting common PHP debugging issues for the description of some common configuration issues and learn how to troubleshoot them.