App Quick Start Guide
Apps are software components that add specific features to YouTrack. With apps, you can customize and extend the base functionality of the system without altering its core structure. This modular approach lets you enhance YouTrack's capabilities dynamically, making it more flexible and adaptable to your organization's needs.
To create an app and start using it in YouTrack, follow these steps:
- Create an app package
- Create the manifest for the app
- Provide a schema of custom settings for your app
- Add a widget
- Add custom HTTP handlers
- Add more modules
- Upload and test the app in YouTrack
- Work with your app
- Publish the app to JetBrains Marketplace
Create an App Package
The fastest way to build a custom widget is to use the YouTrack App Generator. When you run the generator:
The generator builds the basic project structure and installs all the required dependencies.
The prompts in the command line let you specify values for required fields in the
manifest.json
file.The code for a sample widget is saved to the
app
subdirectory.
To generate the code for a new YouTrack app:
Prepare an empty directory for your app.
Using a command line tool, run the following command:
npm create @jetbrains/youtrack-app
Follow the prompts provided by the generator.
Here's an image that shows the file structure of an app package created using the YouTrack App Generator:
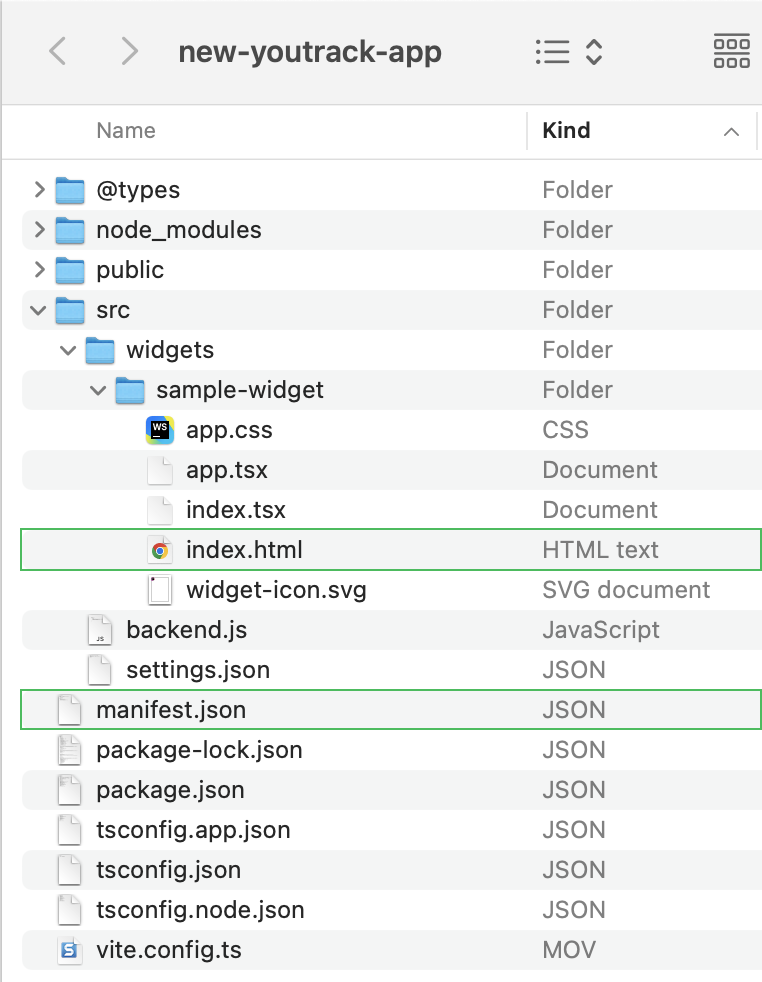
If you happen to want to move files around in the package, please pay attention to the following guidelines for managing the file structure:
The
manifest.json
file must always be present at the root level of the app package.Source code for each widget must be stored in a subdirectory of the
widgets
folder. Thewidgets
folder can be placed at any location in the app package.Each widget must include an
file that defines its content. This file is expected to reside in theindex.html
widget
folder that contains the code for the corresponding widget or one of its subdirectories. Do not storeindex.html
files in parent directories.Store the source code for HTTP handlers and other JavaScript-based modules in separate files at the root level.
Update the App Manifest
Each app package must contain a manifest.json
file. This manifest contains general information about the app and its structure.
For more details about manifests, see App Manifest.
Below, you can find a manifest template that you can modify to define your app structure. This template contains all the fields that are supported for apps in the JetBrains Marketplace.
{
{...}{ "$schema": "https://json.schemastore.org/youtrack-app.json", "name": "template-app", "title": "Template App", "description": "App description", "version": "1.0.0", "url": "https://github.com/example/youtrack-app", "icon": "icon.svg", "iconDark": "icon-dark.svg", "minYouTrackVersion": "2022.2.0", "maxYouTrackVersion": "2024.2.0", "changeNotes": "Version 0.0.1: Feature 1", "vendor": { "name": "JetBrains s.r.o.", "url": "https://www.jetbrains.com/", "email": "support@jetbrains.com" }, "widgets": [ { "key": "sample-widget", "name": "Sample Widget", "description": "Optional widget description", "extensionPoint": "ISSUE_FIELD_PANEL_FIRST", "indexPath": "index.html", "iconPath": "icon.png", "settingsSchemaPath": "sample-widget-settings.json", "permissions": ["ISSUE_READ", "ADMIN_READ_APP"], "defaultDimensions": { "height": "40px", "width": "80%" }, "expectedDimensions": { "height": 40, "width": 150 } } ] }
Provide the Schema for the App Settings
As an app developer, you can provide custom settings for your app. The setting schema is described in the settings.json
file. These settings are then accessible for configuration on the Settings tab of the app.
When a system or a project administrator provides values for the app settings, these values can be used in any JavaScript-based scripts with the app package. For more details about app settings, see App Settings.
Here you can find an example of a settings.json
file:
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "object",
"title": "App Settings",
"description": "Here you can provide values for the app settings",
"properties": {
"stringSetting": {
"title": "Name",
"type": "string",
"minLength": 1
},
"booleanSetting": {
"type": "boolean"
},
"integerSetting": {
"type": "integer",
"x-scope": "GLOBAL"
},
"numberSetting": {
"type": "number",
"x-scope": "PROJECT"
},
"secretSetting": {
"type": "string",
"format": "secret"
},
"arraySetting": {
"type": "array",
"items": {
" x-entity": "User",
"type": "object"
}
},
"userSetting": {
"type": "object",
"x-entity": "User"
}
}
}
Add a Widget
Each app package can contain one or more widgets. The source code for each widget is stored in a named subdirectory of the widgets
folder.
Each widget included in the app package must be declared in the app manifest. The most important part of the description of a widget is the extensionPoint
property. This property specifies the location in the YouTrack UI where the widget will be embedded.
For the complete list of possible extension points in the YouTrack UI, see Extension Points.
A widget is a custom object that you can embed in one of the dedicated extension points in the YouTrack UI. To implement a widget, you need to know the basics of HTML. You can also add custom layout and formatting using CSS.
Here is a sample code for a simple HTML file with the code for a widget displaying the customer logo embedded above the custom field panel in an issue:
<!doctype html>
<html>
<head>
<link rel="stylesheet" href="../index.css">
</head>
<body class="plugin" style="padding: 0; overflow: hidden;">
<img src="logo.jpeg" style="width: 100%; height: 100%; object-fit: cover;"/>
<script type="module">
const host = await YTApp.register();
</script>
</body>
</html>
Add HTTP Handlers
You can create a custom HTTP handler to access YouTrack data from the app through a custom HTTP endpoint. Follow these guidelines when adding an HTTP handler:
Source code for each HTTP handler should be stored in a separate JavaScript file at the root level of the app package.
YouTrack only supports JavaScript as the programming language for the HTTP handlers.
You don't have to add references to the HTTP handlers in the app manifest.
For more information about custom HTTP handlers, see HTTP Handlers.
Here is some sample code for a custom HTTP handler:
exports.httpHandler = {
endpoints: [
{
scope: 'issue',
method: 'GET',
path: 'demo',
handle: function (ctx) {
ctx.response.json({message: "Hello World"});
}
}
]
};
Store this code in a separate sample-http-handler.js
file and place it at the root level of the app package.
To use an HTTP handler, invoke its method from a widget. Here is a sample HTML script block:
<script type="module">
const host = await YTApp.register();
host.alert('Hello world');
</script>
For details about using HTTP handlers, see HTTP Handlers.
Add More Modules
YouTrack supports several JavaScript-based modules that you can add to your app. Here is the list of possible modules:
For more information about available modules, refer to App Reference.
Upload the App to YouTrack
When you're ready to put your app to the test, you can upload it to YouTrack. To test your app, you'll probably want to create a staging environment. You have a couple of options:
Register an instance in YouTrack Cloud. YouTrack Cloud is always updated to the latest available version, which means you can be assured that you're testing in a stable environment with the latest bugfixes.
If your production installation is also hosted by JetBrains, we strongly recommend that you use this environment for testing as well.
Download and run a copy of YouTrack Server on a local machine. If you're working with a YouTrack Server installation, this lets you test your app on the same product version that is currently installed in your production environment.
If you create your app using the YouTrack App Generator, you can use the following commands to build and upload the app to a target YouTrack site:
npm run build
This command compiles the app package and prepares it for upload.
npm run upload -- --host <your YouTrack base URL> --token <permanent token>
This command uploads the app package to the YouTrack site specified as the
host
using the base URL for your YouTrack test environment.For the
permanent token
, you'll need to provide a permanent token that has been generated for the YouTrack account you want to use to upload the app. This account requires Update Project permission for any project in the system. To learn how to generate a permanent token, please refer to the YouTrack documentation.
You can also follow these steps to upload a ZIP archive to your YouTrack site. Since this procedure is done directly in YouTrack by an authenticated user, it doesn't require that you generate a permanent token.
To manually upload an app to YouTrack:
tip
Requires permissions: Update Project
Access your test environment for YouTrack.
From the Administration menu, select Apps.
Click the Add app button and select the Upload ZIP file option.
Select the ZIP archive on your machine and upload it to YouTrack.
The app is added to the list of apps in your YouTrack test environment.
The app and all modules are activated by default.
To check that the app has been uploaded and its modules are active, locate the app in the list and select it.
The Details panel for the selected app opens.
App modules can be built to function at different levels. Some are designed to work at the project level, which means they can be activated independently in different projects. Other modules don't have any connection to a specific project and function at the global level.
Global-level modules are available across the YouTrack system at the moment when the app is imported to YouTrack and activated.
To start using project-level modules, attach the app to a project in YouTrack. You can attach an app to a project either from the Apps administration page or on the Apps tab in the project settings.
For more information about project- and global-level module scopes, see Global and Project Scopes.
To attach an app to a project from the app administration page:
tip
Requires permissions: Update Project
Work with the App
When you have finished testing and updating your app, you're ready to make it available for use inside your organization. Uploading a new app to YouTrack requires that you have the Update Project permission at the global level or the Low-level Admin Write permission in the system. If you have this level of access in your production environment, you can upload and activate the app yourself. Otherwise, you'll need to share your ZIP archive with an administrator who can upload it for you.
Once your app has been uploaded and activated in YouTrack, you and your team can start to enjoy the extended functionality it provides.
Publish the App
The final and optional step is to share your app with other companies who are working with YouTrack by uploading it to JetBrains Marketplace.
To upload your app to the marketplace:
In your web browser, access JetBrains Marketplace.
Click the Sign In button in the header.
If you already have a JetBrains Account, enter your email address and password.
If you don't have a JetBrains Account, register with your email address and create an account.
Select the Build Plugins option in the header.
Click the Upload plugin button.
The Upload plugin page opens.
In the Vendor Details section of the page, select the option to upload the app as an Individual or Organization. When you select the Individual option, you're prompted to create a vendor profile for your account.
In the Plugin Details — Plugin For section of the page, select YouTrack.
For the Plugin file field, click the Choose File button and select the ZIP archive that contains your app package.
For the License select the license you are using to distribute your software.
If your app is open source, enter the location of the repository as the Source code URL.
Use the Tags setting to assign one or more labels to your app. These labels help people find relevant apps in the marketplace.
Click the Upload Plugin button.
Your app is uploaded to the JetBrains Marketplace.
When a moderator has reviewed the app and approved it for publication, the app is added to the directory.